API
If you're looking for an API, you can choose from your desired programming language.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
import requests
api_key = "YOUR_API_KEY"
url = "https://api.segmind.com/v1/flux-pro"
# Prepare data and files
data = {}
files = {}
data['prompt'] = "A mesmerizing 4D rendering illustration masterpiece, capturing the name '1 million users congratulations'under in a captivating, dripping, melting style. The bright, bold white letters are illuminated with vivid hues and emit a whimsical, fun movement. The surrounding abstract shapes, splashes, and drops in various bright colors add to the dynamic atmosphere. The pristine white background contrasts with the bold, striking colors of the text and its surroundings, showcasing the artist's exceptional skills in 3D rendering and illustration. The artist's innovative fusion of typography, 3D rendering, and illustration results in an awe-inspiring piece that is visually stunning, transcending the boundaries of painting, 3D rendering, and vibrant typography., 3d render"
data['guidance'] = 3
data['steps'] = 25
data['interval'] = 2
data['safety_tolerance'] = 2
data['aspect_ratio'] = "1:1"
data['seed'] = 46588
data['output_format'] = "webp"
data['output_quality'] = 80
headers = {'x-api-key': api_key}
# If no files, send as JSON
if files:
response = requests.post(url, data=data, files=files, headers=headers)
else:
response = requests.post(url, json=data, headers=headers)
print(response.content) # The response is the generated image
Attributes
Text prompt for image generation
guidance
min : 2,
max : 5
number of steps
min : 1,
max : 50
interval
min : 1,
max : 4
safety tolerance
min : 1,
max : 5
Type of scheduler.
Allowed values:
Seed for random number generation
An enumeration.
Allowed values:
Quality when saving the output images, from 0 to 100. 100 is best quality, 0 is lowest quality. Not relevant for .png outputs
min : 0,
max : 100
To keep track of your credit usage, you can inspect the response headers of each API call. The x-remaining-credits property will indicate the number of remaining credits in your account. Ensure you monitor this value to avoid any disruptions in your API usage.
Flux .1 Pro
Flux Pro by Black Forest Labs represents the pinnacle of text-to-image generative models, leveraging a sophisticated hybrid architecture that combines multimodal and parallel diffusion transformer blocks. Scaled to 12 billion parameters, Flux Pro enhances previous state-of-the-art diffusion models using flow matching, a versatile and conceptually straightforward method for training generative models. Additionally, the model incorporates rotary positional embeddings and parallel attention layers, significantly improving performance and hardware efficiency.
How to Use the Model
To use the Flux Pro model:
-
Input Text Prompt: Provide a textual description of the desired image. The model processes this input to generate a corresponding visual output.
-
Run the Model: Execute the model with your text input. The AI algorithm interprets the description to produce an image.
-
Review Outputs: Evaluate the generated images for quality and relevance to your input.
Use Cases
-
Graphic Design: Automate the creation of graphics based on simple text descriptions, saving time on repetitive design tasks.
-
Advertising: Generate visual content tailored to marketing campaigns, quickly producing assets that align with brand messages.
-
Content Creation: Assist writers and content creators in visualizing their narratives by generating illustrative images from textual descriptions.
-
Web Development: Enhance websites with unique, dynamically generated images that improve user engagement and aesthetic appeal.
-
Research and Development: Utilize the model for experimental purposes in AI research, testing the boundaries of text-to-image generation capabilities.
Other Popular Models
idm-vton
Best-in-class clothing virtual try on in the wild

faceswap-v2
Take a picture/gif and replace the face in it with a face of your choice. You only need one image of the desired face. No dataset, no training
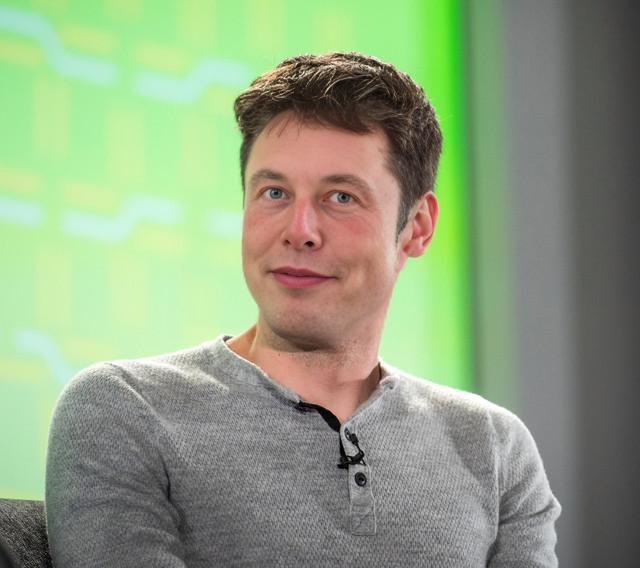
sdxl-inpaint
This model is capable of generating photo-realistic images given any text input, with the extra capability of inpainting the pictures by using a mask
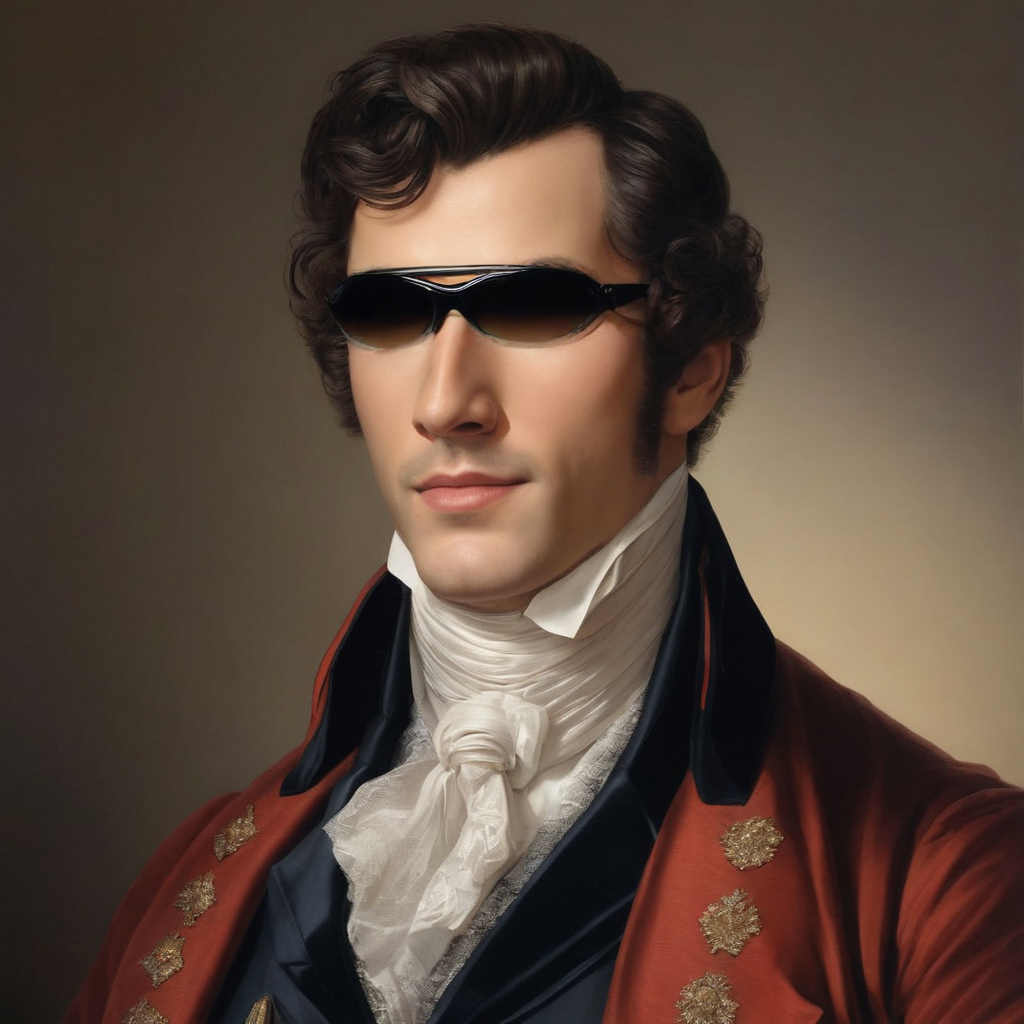
sd2.1-faceswapper
Take a picture/gif and replace the face in it with a face of your choice. You only need one image of the desired face. No dataset, no training
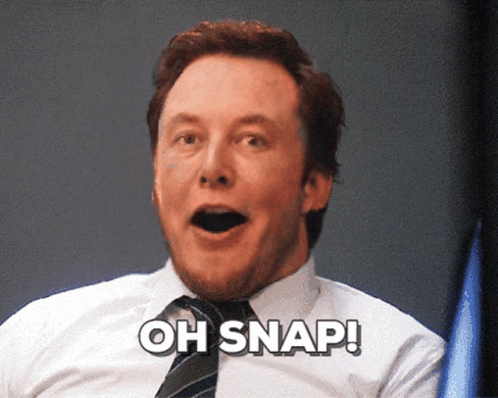