API
If you're looking for an API, you can choose from your desired programming language.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
import requests
import base64
# Use this function to convert an image file from the filesystem to base64
def image_file_to_base64(image_path):
with open(image_path, 'rb') as f:
image_data = f.read()
return base64.b64encode(image_data).decode('utf-8')
# Use this function to fetch an image from a URL and convert it to base64
def image_url_to_base64(image_url):
response = requests.get(image_url)
image_data = response.content
return base64.b64encode(image_data).decode('utf-8')
# Use this function to convert a list of image URLs to base64
def image_urls_to_base64(image_urls):
return [image_url_to_base64(url) for url in image_urls]
api_key = "YOUR_API_KEY"
url = "https://api.segmind.com/v1/focus-inpaint"
# Request payload
data = {
"prompt": "Photo of a car on a road in a hill station",
"negative_prompt": "lowquality, badquality, sketches",
"steps": 30,
"samples": 1,
"styles": [
"Fooocus V2",
"Fooocus Sharp",
"Fooocus Enhance"
],
"aspect_ratios": "1024*1024",
"seed": 354849415,
"guidance_scale": 4,
"scheduler": "karras",
"base_model": "juggernaut_v8",
"sampler": "dpmpp_2m_sde_gpu",
"input_image": image_url_to_base64("https://segmind-sd-models.s3.amazonaws.com/display_images/focus-inpaint-input.jpg"), # Or use image_file_to_base64("IMAGE_PATH")
"input_mask": image_url_to_base64("https://segmind-sd-models.s3.amazonaws.com/display_images/focus-inpaint-mask.jpg"), # Or use image_file_to_base64("IMAGE_PATH")
"inpaint_erode_or_dilate": 1,
"inpaint_respective_field": 0.618,
"inpaint_strength": 1,
"invert_mask_checkbox": "False",
"mixing_image_prompt_and_inpaint": "True",
"faceswap_cn_stop": 0.9,
"faceswap_cn_weight": 0.8,
"imageprompt_cn_stop": 0.5,
"imageprompt_cn_weight": 0.6,
"pyracanny_cn_stop": 0.5,
"pyracanny_cn_weight": 1,
"cpds_cn_stop": 0.5,
"cpds_cn_weight": 1,
"base64": False
}
headers = {'x-api-key': api_key}
response = requests.post(url, json=data, headers=headers)
print(response.content) # The response is the generated image
Attributes
Prompt to render
Prompts to exclude, eg. bad anatomy, bad hands, missing fingers
Number of denoising steps.
min : 20,
max : 100
Number images to generate.
min : 1,
max : 4
Style selection
Output image aspect ratio
Allowed values:
Additional Prompt for Inpainting
Seed for image generation.
min : -1,
max : 999999999999999
Scale for classifier-free guidance
min : 1,
max : 25
Type of scheduler.
Allowed values:
Base model for inference
Allowed values:
Type of sampler
Allowed values:
Input image
Input Mask
Erode or Dilate values. Negative implies Erode and vice versa
min : -50,
max : 50
Inpaint Respective Field
min : 0,
max : 1
Inpaint strength
min : 0,
max : 1
Invert mask checkbox
Mixing image prompt and inpaint
Face image for swapping
Face swap stop value
min : 0,
max : 1.5
Face swap weight value
min : 0,
max : 1.5
Image prompt image
Ip controlnet stop value
min : 0,
max : 1.5
Ip controlnet weight
min : 0,
max : 1.5
Pyracanny image
Controlnet stop value
min : 0,
max : 1.5
Controlnet weight value
min : 0,
max : 1.5
CPDS image
Controlnet stop value
min : 0,
max : 1.5
Controlnet weight value
min : 0,
max : 1.5
Base64 encoding of the output image.
To keep track of your credit usage, you can inspect the response headers of each API call. The x-remaining-credits property will indicate the number of remaining credits in your account. Ensure you monitor this value to avoid any disruptions in your API usage.
Fooocus Inpainting
Fooocus Inpainting is a powerful image generation model that allows you to selectively edit and enhance images. Whether you’re restoring old photographs, removing unwanted objects, or filling in missing regions, Fooocus Inpainting provides a versatile solution.
-
Input Image: Please upload the image you’d like to inpaint. Make sure it’s in a common image format (such as JPEG or PNG). If there are specific regions you want to inpaint, consider providing a mask as well.
-
Mask: If you have a mask indicating the areas that need inpainting (e.g., the scratched portion of an old photo), please upload it. The mask helps guide the inpainting process.
-
Text Prompt: Describe your desired outcome or any specific instructions related to the inpainting. For example, if you want to restore missing parts of an old family portrait, mention that.
When working with Fooocus Inpainting, providing reference images and using specific techniques can indeed enhance your control over image manipulation. Here’s how you can leverage these tools:
Reference Images for Image Prompt: If you have specific visual references or examples of the desired inpainting outcome, consider including them. These reference images can guide the model by providing context, style, and content cues.
Pyracanny: Pyracanny is a powerful edge detection technique. By applying Pyracanny to your input image, you can extract precise edge information. Use this edge map as an additional input to Fooocus Inpainting. It helps the model understand object boundaries and ensures smoother transitions between inpainted and original regions.
Face Image: This is a specific kind of image prompt where the provided image focuses on a face. It’s useful when you want to specifically modify or generate an image centered around a face.
CPDS (Contour-Preserved Dense Sampling): CPDS is a technique that preserves contours during image manipulation. If you want to maintain sharp edges or specific contours (e.g., architectural details, tree branches, or intricate patterns), use CPDS. It ensures that the inpainted regions blend seamlessly while retaining essential features.
Other Popular Models
sdxl-img2img
SDXL Img2Img is used for text-guided image-to-image translation. This model uses the weights from Stable Diffusion to generate new images from an input image using StableDiffusionImg2ImgPipeline from diffusers
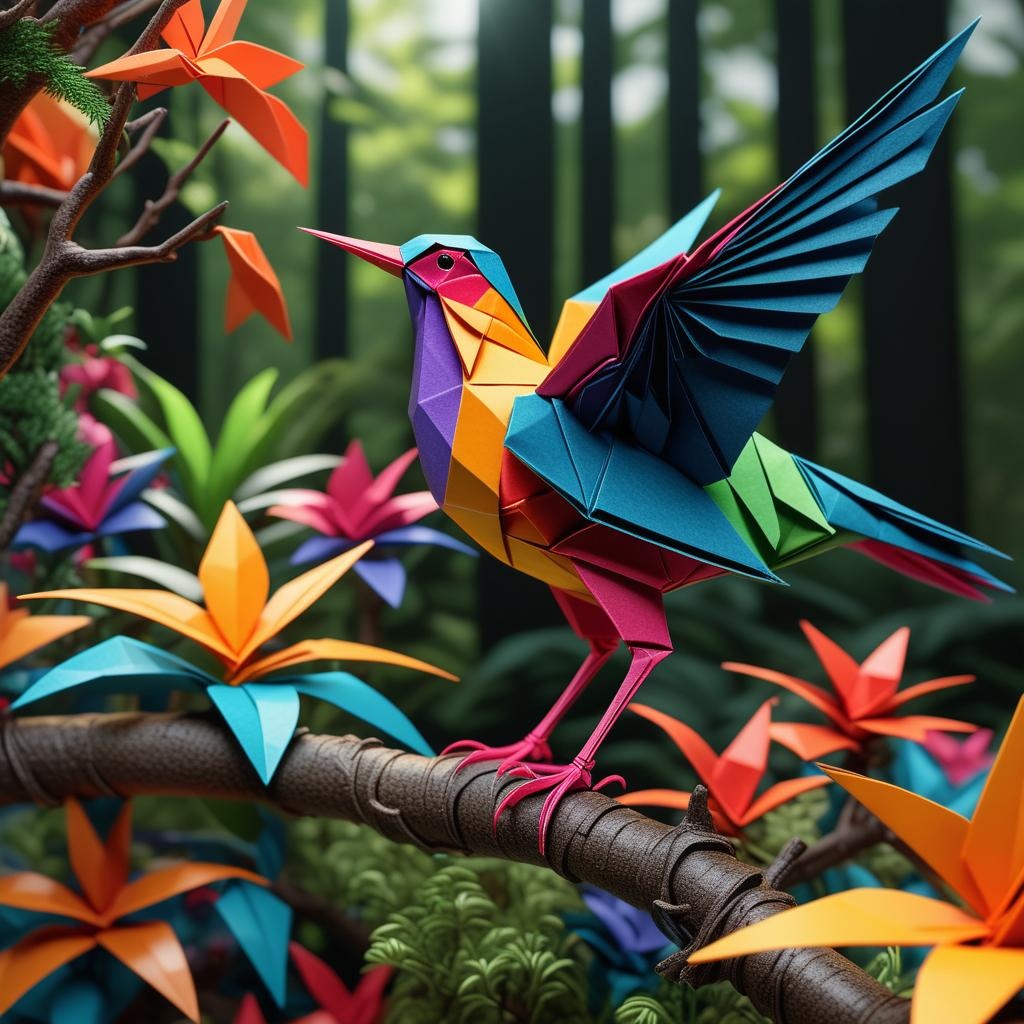
idm-vton
Best-in-class clothing virtual try on in the wild

faceswap-v2
Take a picture/gif and replace the face in it with a face of your choice. You only need one image of the desired face. No dataset, no training
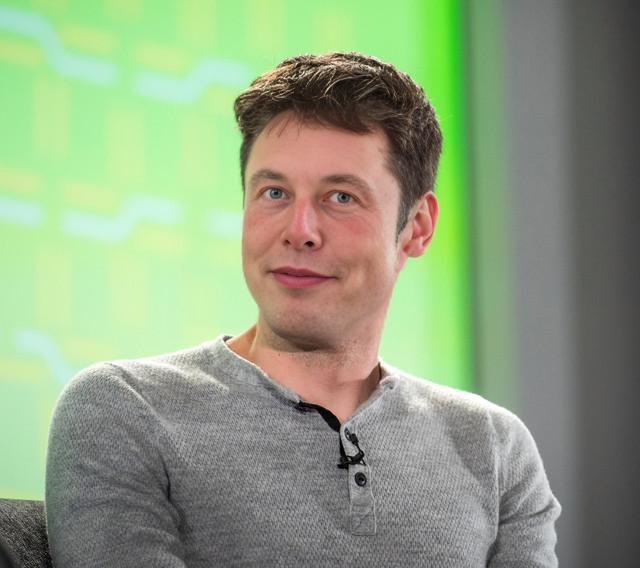
sdxl1.0-txt2img
The SDXL model is the official upgrade to the v1.5 model. The model is released as open-source software
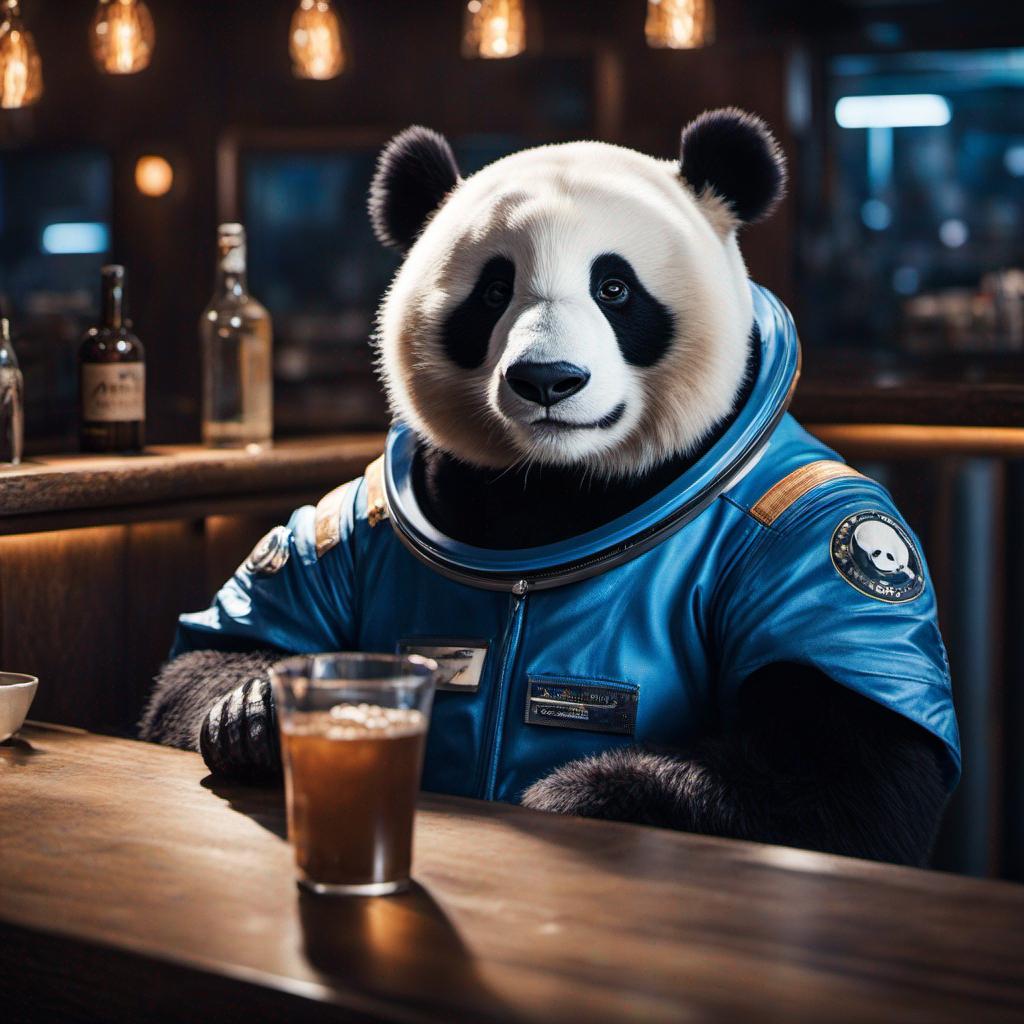