API
If you're looking for an API, you can choose from your desired programming language.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
import requests
import base64
# Use this function to convert an image file from the filesystem to base64
def image_file_to_base64(image_path):
with open(image_path, 'rb') as f:
image_data = f.read()
return base64.b64encode(image_data).decode('utf-8')
# Use this function to fetch an image from a URL and convert it to base64
def image_url_to_base64(image_url):
response = requests.get(image_url)
image_data = response.content
return base64.b64encode(image_data).decode('utf-8')
# Use this function to convert a list of image URLs to base64
def image_urls_to_base64(image_urls):
return [image_url_to_base64(url) for url in image_urls]
api_key = "YOUR_API_KEY"
url = "https://api.segmind.com/v1/focus-outpaint"
# Request payload
data = {
"prompt": "Photo of man standing in a street",
"negative_prompt": "lowquality, badquality, sketches",
"steps": 30,
"samples": 1,
"styles": "V2,Enhance,Sharp",
"aspect_ratios": "1024*1024",
"seed": 354849415,
"guidance_scale": 4,
"scheduler": "karras",
"sampler": "dpmpp_2m_sde_gpu",
"base_model": "juggernaut_v8",
"input_image": image_url_to_base64("https://segmind-sd-models.s3.amazonaws.com/display_images/focus-outpaint-input.png"), # Or use image_file_to_base64("IMAGE_PATH")
"outpaint_selections": "Right, Left, Top, Bottom",
"base64": False
}
headers = {'x-api-key': api_key}
response = requests.post(url, json=data, headers=headers)
print(response.content) # The response is the generated image
Attributes
Prompt to render
Prompts to exclude, eg. bad anatomy, bad hands, missing fingers
Number of denoising steps.
min : 20,
max : 100
Number images to generate.
min : 1,
max : 4
Style selection
output image aspect ratio
Allowed values:
Seed for image generation.
min : -1,
max : 999999999999999
Scale for classifier-free guidance
min : 1,
max : 25
Type of scheduler.
Allowed values:
Type of sampler.
Allowed values:
Model for inference
Allowed values:
input image
Outpaint Selections
Base64 encoding of the output image.
To keep track of your credit usage, you can inspect the response headers of each API call. The x-remaining-credits property will indicate the number of remaining credits in your account. Ensure you monitor this value to avoid any disruptions in your API usage.
Fooocus Outpainting
Fooocus Outpainting transforms ordinary images into extraordinary works of art by seamlessly expanding their boundaries. Whether you’re a photographer, designer, or digital artist, Fooocus Outpainting equips you with the tools needed to achieve stunning results and take your projects to new heights.
Using Fooocus Outpointing is straightforward:
-
Upload your image: Simply drag and drop your PNG, JPG, or GIF file (up to 2048 x 2048 px) into the input field.
-
Adjust settings: Select your preferred aspect ratio and set your seed. The 'Randomise Seed' option can inject variation for more dynamic results.
-
Choose expansion directions: Specify the areas where you wish to expand your image - right, left, top, bottom, or a combination thereof.
-
Generate: Click the 'Generate' button to process your image. In moments, Fooocus Outpainting produces an expanded and refined version of your original image.
Fooocus Outpainting is ideal for a myriad of applications:
-
Photography: Enhance landscape photos by extending the horizons or adding elements to the composition.
-
Product Design: Improve visual presentations by expanding the background to accommodate textual or graphic elements.
-
Digital Art: Experiment with new compositions and perspectives, pushing the boundaries of your creativity.
-
Marketing and Advertising: Create comprehensive visuals for campaigns, ensuring every image captivates your audience.
Other Popular Models
sdxl-controlnet
SDXL ControlNet gives unprecedented control over text-to-image generation. SDXL ControlNet models Introduces the concept of conditioning inputs, which provide additional information to guide the image generation process
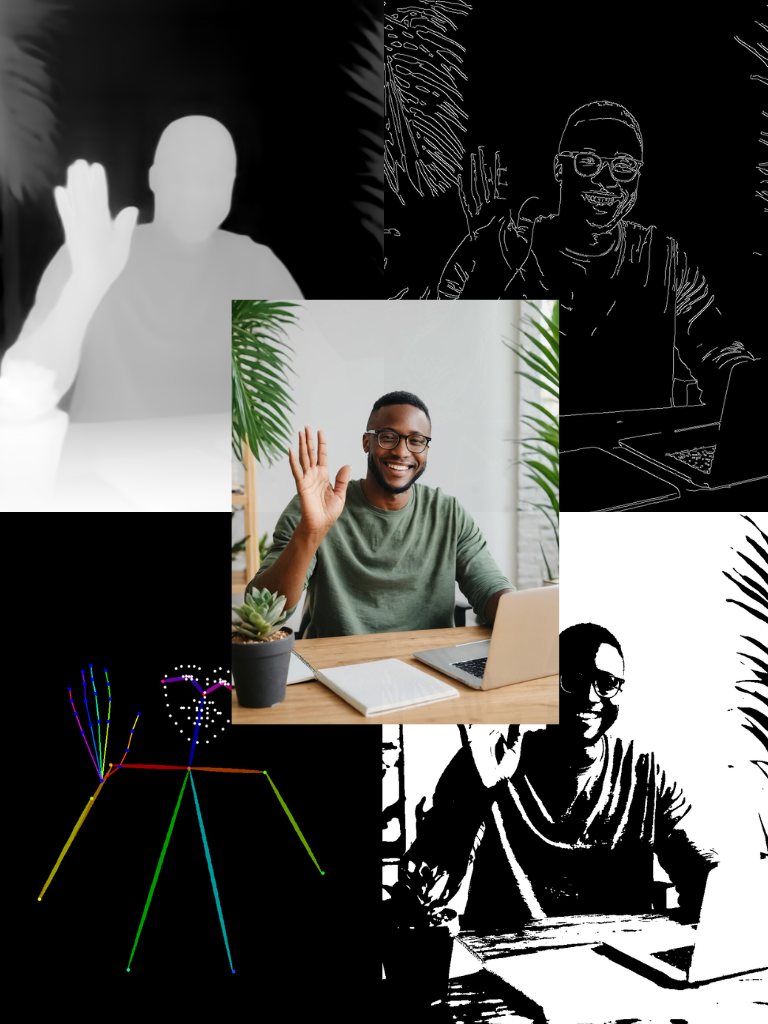
fooocus
Fooocus enables high-quality image generation effortlessly, combining the best of Stable Diffusion and Midjourney.
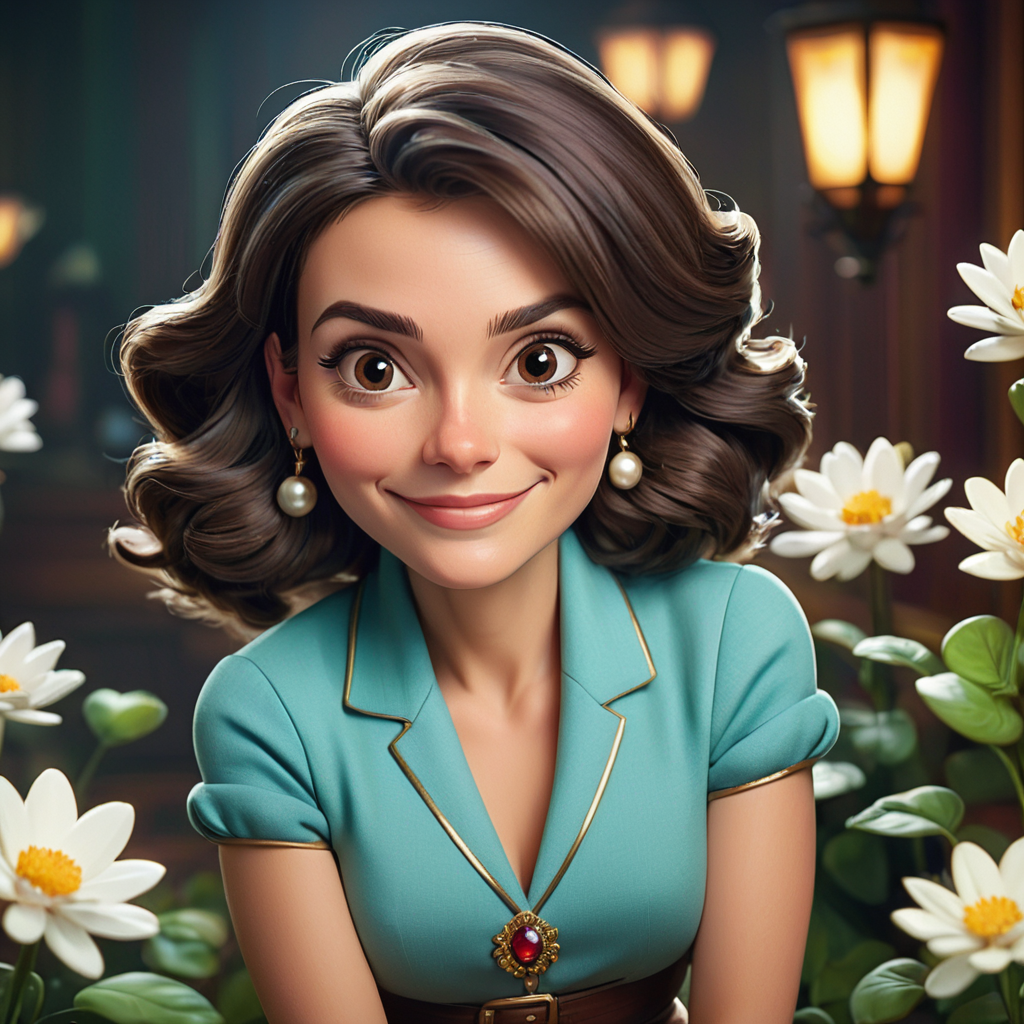
sd1.5-majicmix
The most versatile photorealistic model that blends various models to achieve the amazing realistic images.
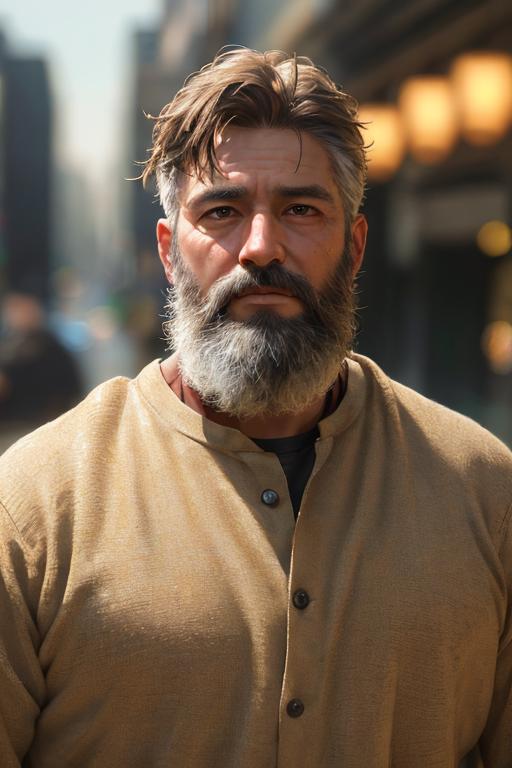
codeformer
CodeFormer is a robust face restoration algorithm for old photos or AI-generated faces.
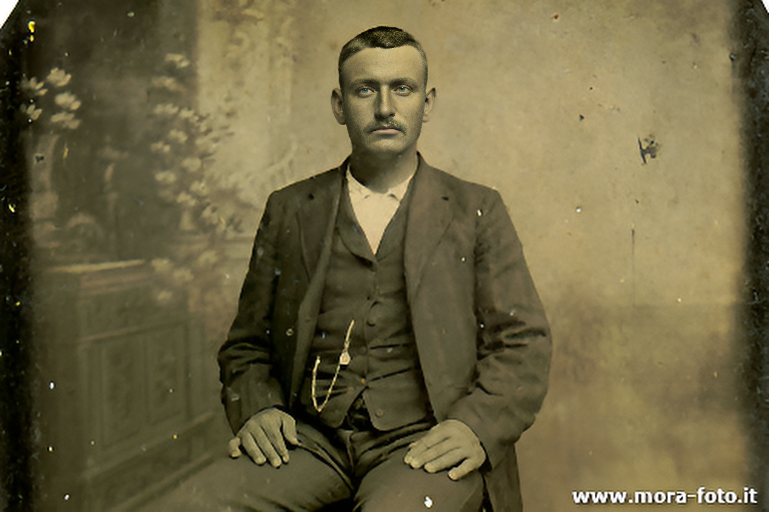