GPT 4 turbo
GPT-4 outperforms both previous large language models and as of 2023, most state-of-the-art systems (which often have benchmark-specific training or hand-engineering). On the MMLU benchmark, an English-language suite of multiple-choice questions covering 57 subjects, GPT-4 not only outperforms existing models by a considerable margin in English, but also demonstrates strong performance in other languages. Currently points to gpt-4-turbo-2024-04-09.
API
If you're looking for an API, you can choose from your desired programming language.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
const axios = require('axios');
const api_key = "YOUR API-KEY";
const url = "https://api.segmind.com/v1/gpt-4-turbo";
const data = {
"messages": [
{
"role": "user",
"content": "tell me a joke on cats"
},
{
"role": "assistant",
"content": "here is a joke about cats..."
},
{
"role": "user",
"content": "now a joke on dogs"
}
]
};
(async function() {
try {
const response = await axios.post(url, data, { headers: { 'x-api-key': api_key } });
console.log(response.data);
} catch (error) {
console.error('Error:', error.response.data);
}
})();
Attributes
An array of objects containing the role and content
Could be "user", "assistant" or "system".
A string containing the user's query or the assistant's response.
To keep track of your credit usage, you can inspect the response headers of each API call. The x-remaining-credits property will indicate the number of remaining credits in your account. Ensure you monitor this value to avoid any disruptions in your API usage.
GPT- 4 turbo
GPT-4 Turbo is an advanced system that can retain more information and has knowledge of events that occurred up to April 2023. This is a significant improvement from prior GPT generations, which had a knowledge cut-off of September 2021.
GPT-4 Turbo introduces a 128k context window, equivalent to 300 pages of text in a single prompt. This allows for use cases like long-form content creation, extended conversations, and document search and analysis. It can also solve difficult problems with greater accuracy, thanks to its broader general knowledge and problem-solving abilities.
One of the key features of GPT-4 Turbo is its cost-effectiveness. The model is 3X cheaper for input tokens and 2X cheaper for output tokens compared to the original GPT-4 model.
Other Popular Models
insta-depth
InstantID aims to generate customized images with various poses or styles from only a single reference ID image while ensuring high fidelity
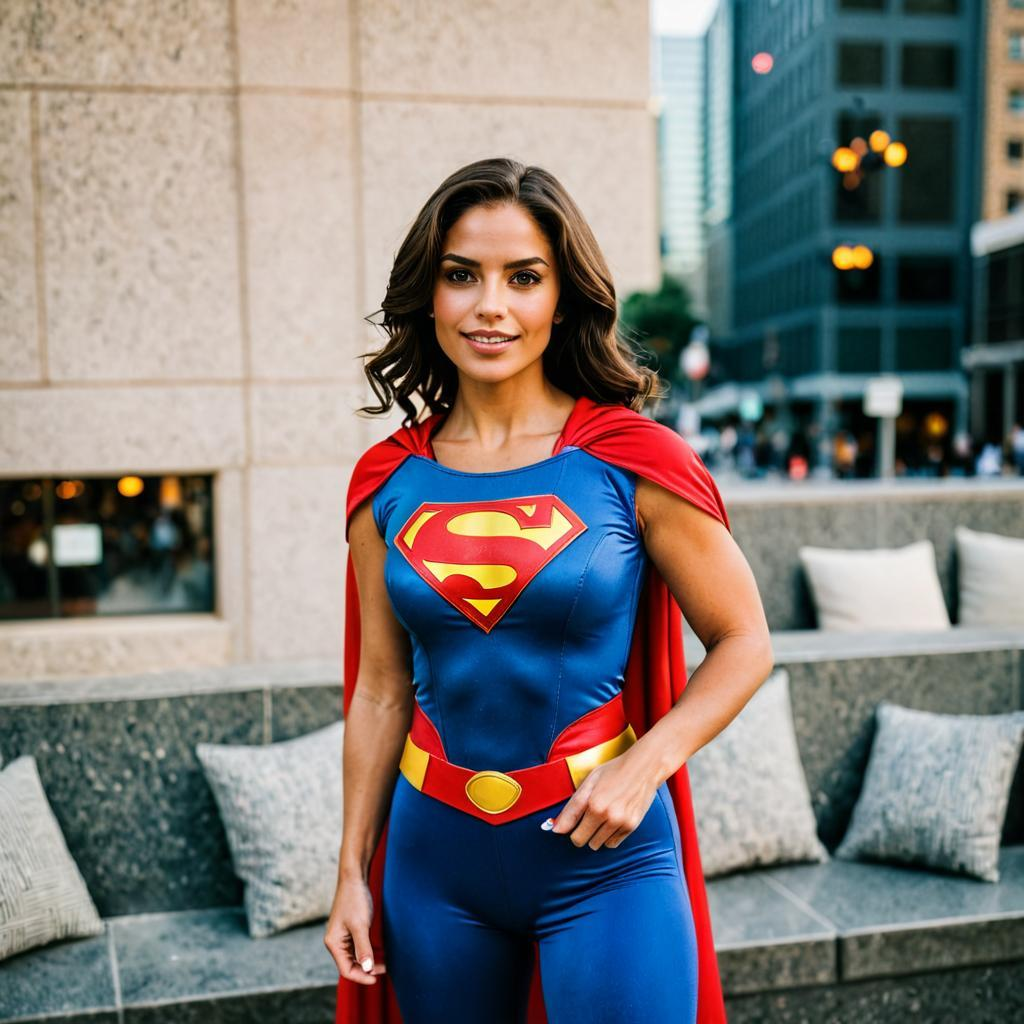
sdxl1.0-txt2img
The SDXL model is the official upgrade to the v1.5 model. The model is released as open-source software
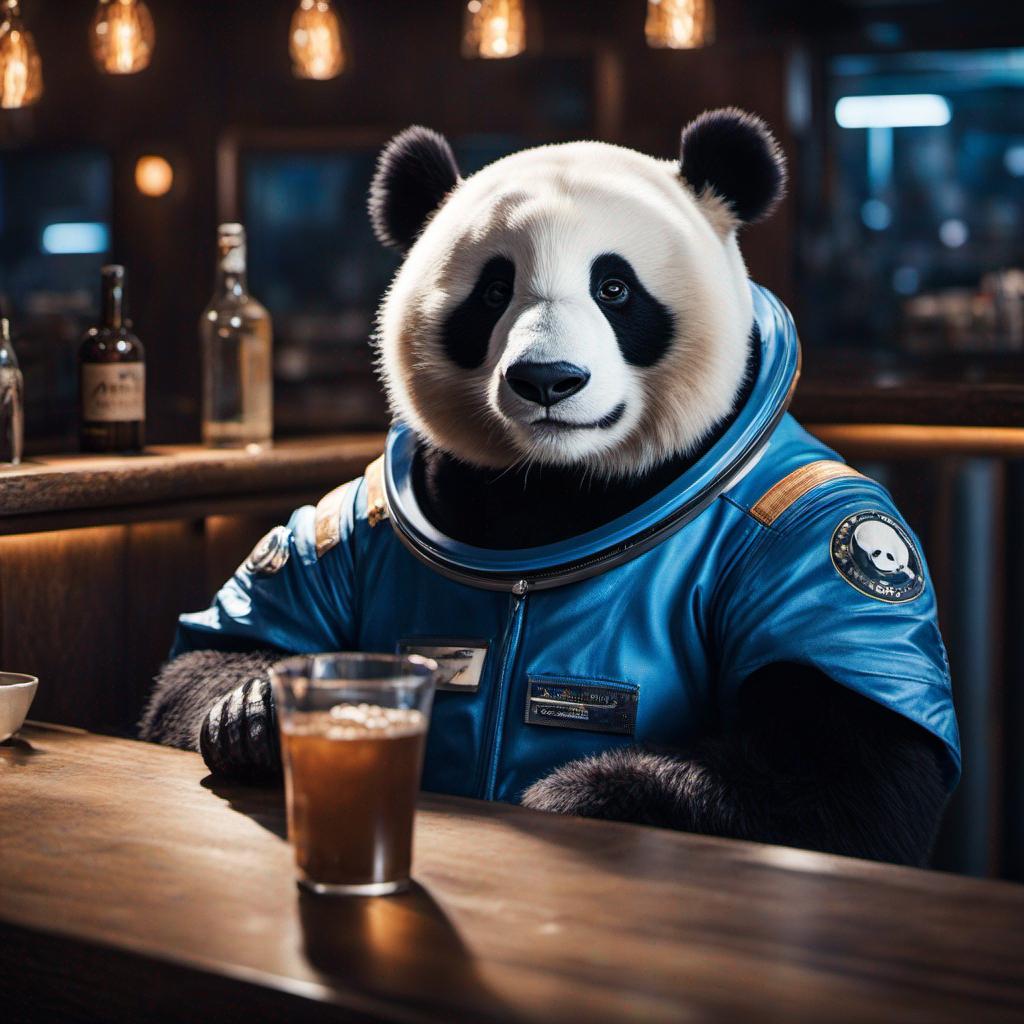
codeformer
CodeFormer is a robust face restoration algorithm for old photos or AI-generated faces.
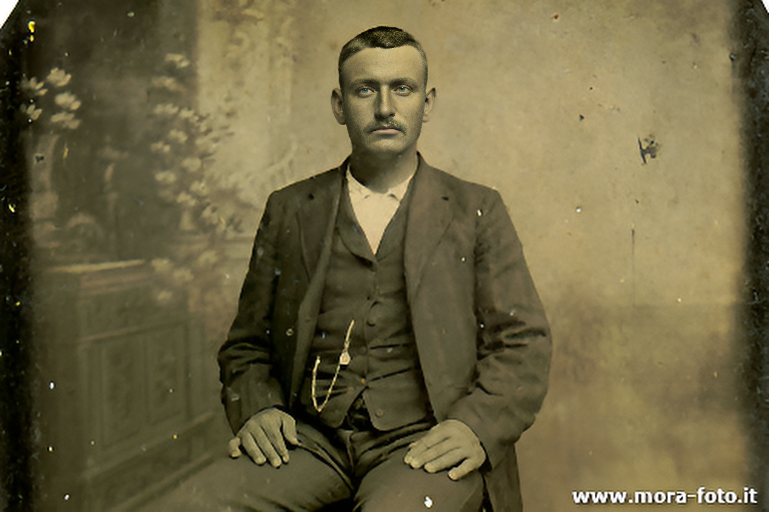
sd2.1-faceswapper
Take a picture/gif and replace the face in it with a face of your choice. You only need one image of the desired face. No dataset, no training
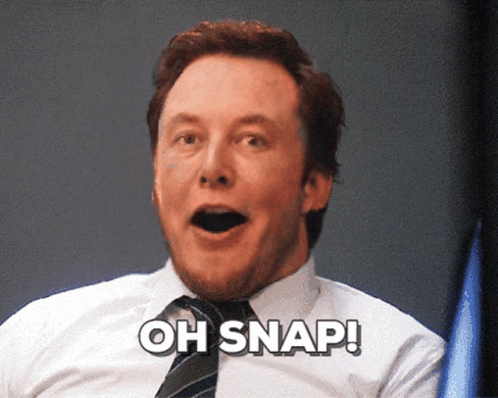