API
If you're looking for an API, you can choose from your desired programming language.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
import requests
import base64
# Use this function to convert an image file from the filesystem to base64
def image_file_to_base64(image_path):
with open(image_path, 'rb') as f:
image_data = f.read()
return base64.b64encode(image_data).decode('utf-8')
# Use this function to fetch an image from a URL and convert it to base64
def image_url_to_base64(image_url):
response = requests.get(image_url)
image_data = response.content
return base64.b64encode(image_data).decode('utf-8')
# Use this function to convert a list of image URLs to base64
def image_urls_to_base64(image_urls):
return [image_url_to_base64(url) for url in image_urls]
api_key = "YOUR_API_KEY"
url = "https://api.segmind.com/v1/ideogram-2a-txt-2-img"
# Request payload
data = {
"magic_prompt_option": "AUTO",
"prompt": "A dreamy, ultra-detailed miniature scene featuring tiny chocolatiers in brown aprons and chef hats, crafting luxurious chocolate treats. Some temper glossy chocolate on a marble slab, while others dip delicate strawberries into molten chocolate fountains. One chef meticulously carves intricate designs into a chocolate bar. The background is a charming chocolate boutique with golden packaging and shelves lined with handcrafted truffles. The rich, warm lighting enhances the decadent and magical atmosphere",
"resolution": "RESOLUTION_1024_1024",
"seed": 56698,
"style_type": "GENERAL"
}
headers = {'x-api-key': api_key}
response = requests.post(url, json=data, headers=headers)
print(response.content) # The response is the generated image
Attributes
Option for magic prompt
Allowed values:
Prompt to render
Resolution of the output image
Allowed values:
Seed for random generation
min : 1,
max : 99999
Style type for the output
Allowed values:
To keep track of your credit usage, you can inspect the response headers of each API call. The x-remaining-credits property will indicate the number of remaining credits in your account. Ensure you monitor this value to avoid any disruptions in your API usage.
Ideogram 2a Text-to-Image
Ideogram 2a text-to-image can turn your ideas into stunning images with a focus on captivating designs, realistic images, and innovative logos. It is particularly useful because of its unique capabilities like text rendering in images. It is aimed to inspire creativity and help every user bring their imagination to life.
Key Features
-
Text Rendering: Excels at incorporating text seamlessly and creatively within generated images.
-
Versatile Image Creation: Create captivating designs, realistic images, and innovative logos.
-
Aspect Ratios & Resolutions: Offers a variety of aspect ratios and resolutions to suit different creative needs.
-
Style Options: Offers a diverse range of artistic styles, from realistic to abstract
Use Cases
-
Logo and Branding Design: Generate unique and memorable logos with integrated text for businesses and personal brands.
-
Marketing and Advertising: Create eye-catching visuals with compelling text for social media campaigns, website banners, and print ads.
-
Content Creation: Produce illustrative images and diagrams with clear text labels for blog posts, articles, and educational materials.
-
Personalized Gifts and Merchandise: Design custom images with text for t-shirts, mugs, posters, and other products.
Other Popular Models
faceswap-v2
Take a picture/gif and replace the face in it with a face of your choice. You only need one image of the desired face. No dataset, no training
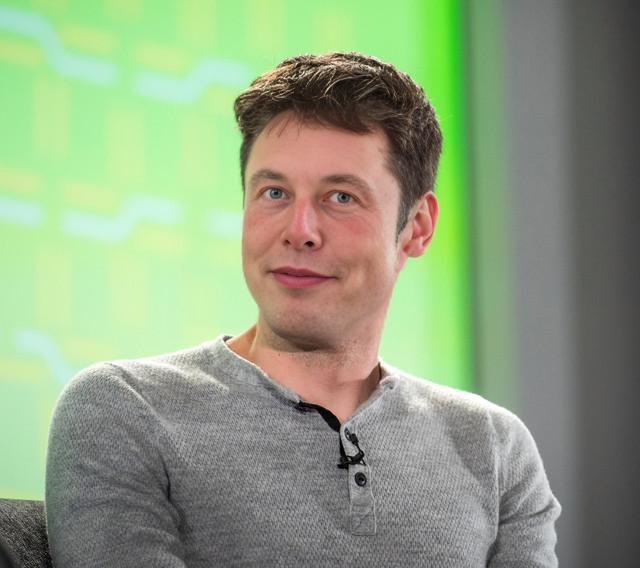
sdxl1.0-txt2img
The SDXL model is the official upgrade to the v1.5 model. The model is released as open-source software
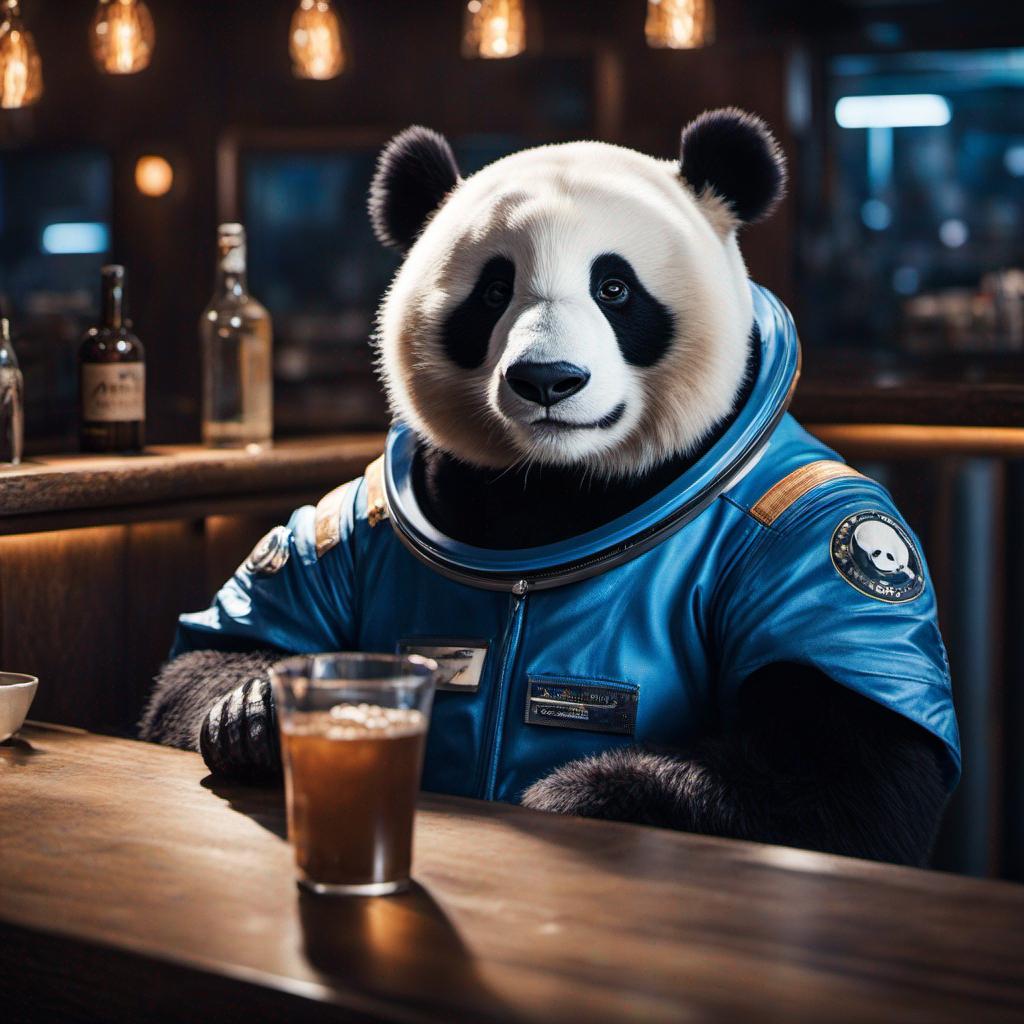
codeformer
CodeFormer is a robust face restoration algorithm for old photos or AI-generated faces.
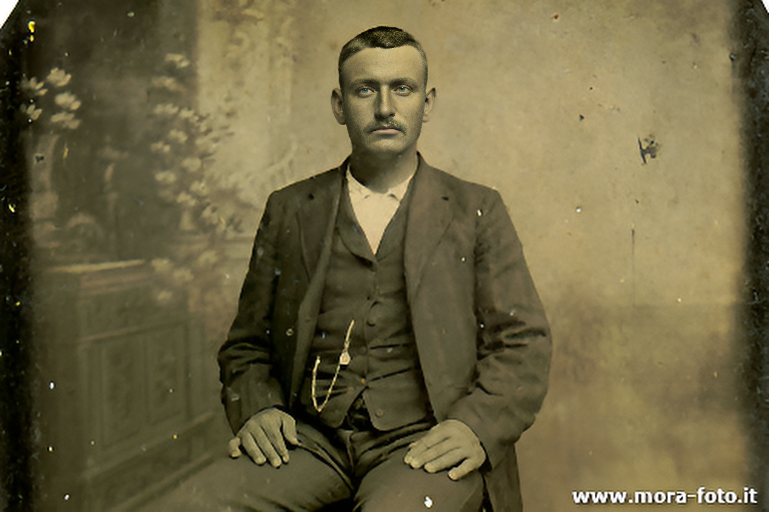
sd2.1-faceswapper
Take a picture/gif and replace the face in it with a face of your choice. You only need one image of the desired face. No dataset, no training
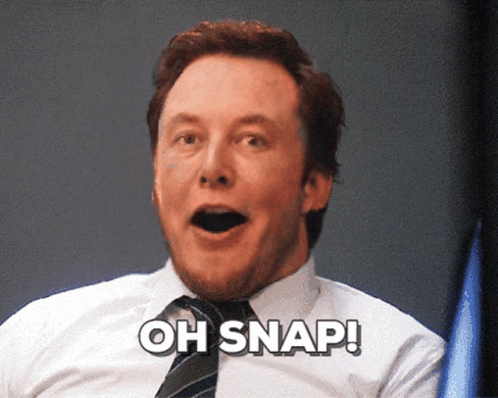