API
If you're looking for an API, you can choose from your desired programming language.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
const axios = require('axios');
const api_key = "YOUR API-KEY";
const url = "https://api.segmind.com/v1/o1-mini";
const data = {
"messages": [
{
"role": "user",
"content": "tell me a joke on cats"
},
{
"role": "assistant",
"content": "here is a joke about cats..."
},
{
"role": "user",
"content": "now a joke on dogs"
}
]
};
(async function() {
try {
const response = await axios.post(url, data, { headers: { 'x-api-key': api_key } });
console.log(response.data);
} catch (error) {
console.error('Error:', error.response.data);
}
})();
Attributes
An array of objects containing the role and content
Could be "user", "assistant" or "system".
A string containing the user's query or the assistant's response.
To keep track of your credit usage, you can inspect the response headers of each API call. The x-remaining-credits property will indicate the number of remaining credits in your account. Ensure you monitor this value to avoid any disruptions in your API usage.
OpenAI o1-mini
o1-mini by OpenAI is a compact yet powerful AI model designed to deliver cost-efficient reasoning capabilities. This model is tailored for developers and businesses seeking high performance without the high costs, making advanced AI accessible to a broader audience.
Key Features of OpenAI o1-mini
-
Cost-Effective Performance: The o1-mini model is engineered to be 80% more cost-efficient than the o1-preview, providing a budget-friendly option for complex reasoning tasks without compromising on quality.
-
Optimized for Coding: Specially designed for coding applications, o1-mini excels in generating, debugging, and optimizing complex code efficiently, making it an invaluable tool for developers.
-
Enhanced Reasoning: Despite its smaller size, o1-mini maintains robust reasoning abilities, making it ideal for tasks that require precise problem-solving and logical analysis.
-
Scalable Solutions: The o1-mini model is perfect for scalable solutions, allowing businesses to integrate advanced AI capabilities into their operations without significant financial investment.
How It Works
The o1-mini model leverages the same advanced reasoning techniques as its larger counterpart, the o1-preview. It is trained to methodically think through problems, ensuring accurate and reliable outputs. This approach allows the model to refine its strategies, recognize mistakes, and deliver more precise results, making it a valuable tool for developers and businesses alike.
The o1-mini incorporates rigorous safety training, ensuring it adheres to strict guidelines and minimizes risks. This model is designed to handle sensitive tasks with care, providing peace of mind for users. It scored impressively on our internal safety benchmarks, demonstrating its reliability and robustness. It integrates seamlessly into existing workflows, offering a versatile solution for various applications. Whether you’re developing new software, enhancing existing systems, or exploring innovative AI applications, o1-mini provides the power you need at a fraction of the cost.
Use Cases
-
Software Development: Enhance your development process with o1-mini’s advanced coding capabilities, from generating boilerplate code to debugging complex algorithms.
-
Data Analysis: Utilize o1-mini for sophisticated data analysis tasks, enabling more accurate and insightful interpretations of large datasets.
-
Customer Support: Implement o1-mini in customer support systems to provide intelligent, automated responses, improving efficiency and customer satisfaction.
-
Research and Development: Leverage o1-mini’s reasoning abilities for research projects, enabling more thorough and accurate analysis of complex problems.
Other Popular Models
storydiffusion
Story Diffusion turns your written narratives into stunning image sequences.
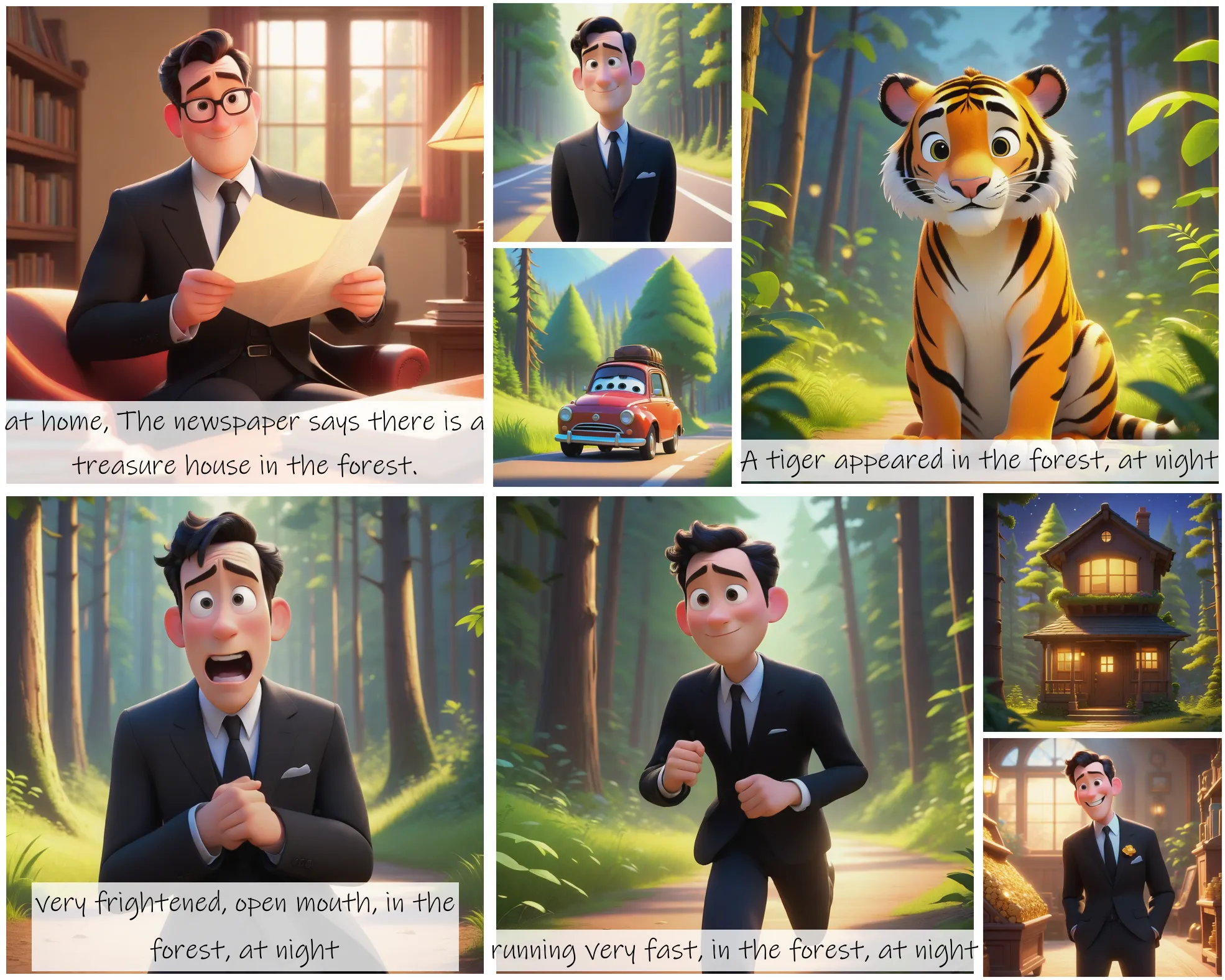
fooocus
Fooocus enables high-quality image generation effortlessly, combining the best of Stable Diffusion and Midjourney.
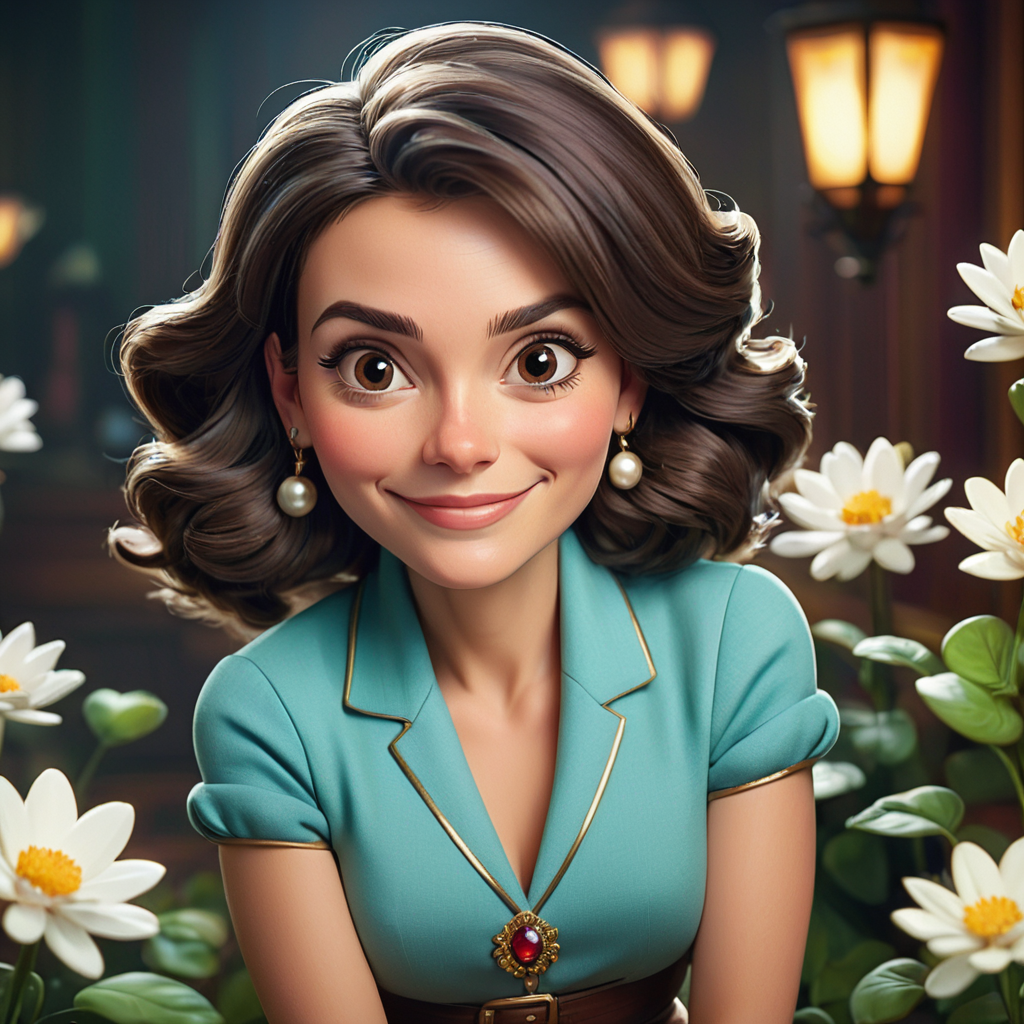
sd1.5-majicmix
The most versatile photorealistic model that blends various models to achieve the amazing realistic images.
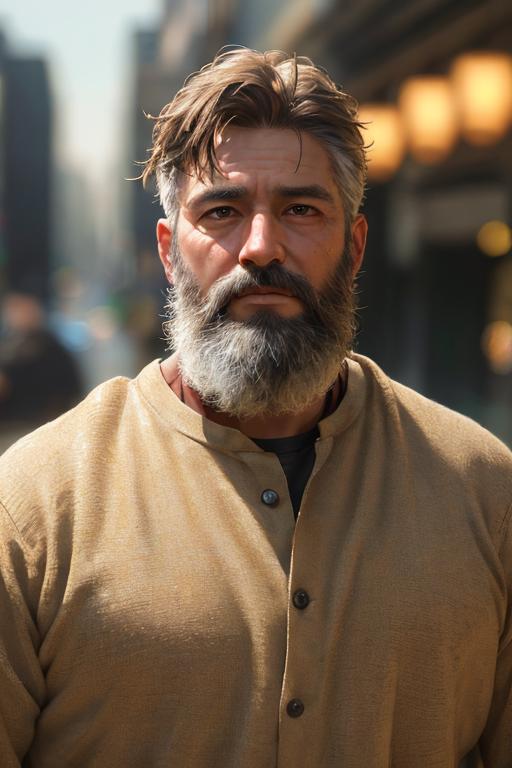
codeformer
CodeFormer is a robust face restoration algorithm for old photos or AI-generated faces.
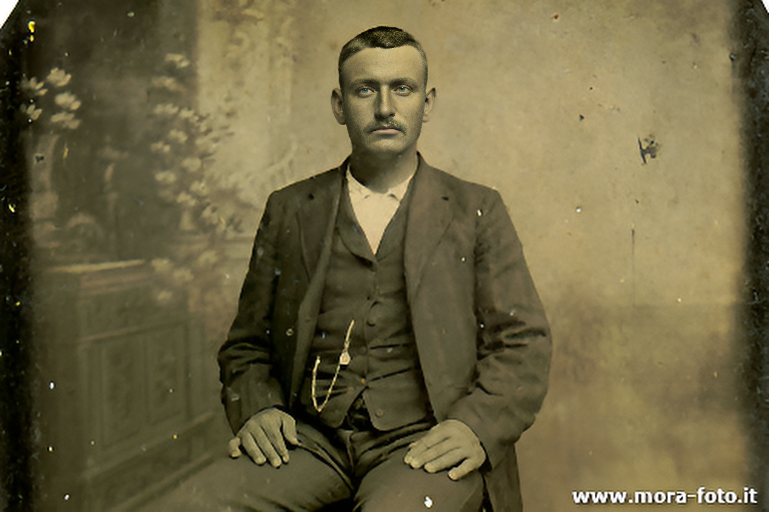