API
If you're looking for an API, you can choose from your desired programming language.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
import requests
import base64
# Use this function to convert an image file from the filesystem to base64
def image_file_to_base64(image_path):
with open(image_path, 'rb') as f:
image_data = f.read()
return base64.b64encode(image_data).decode('utf-8')
# Use this function to fetch an image from a URL and convert it to base64
def image_url_to_base64(image_url):
response = requests.get(image_url)
image_data = response.content
return base64.b64encode(image_data).decode('utf-8')
# Use this function to convert a list of image URLs to base64
def image_urls_to_base64(image_urls):
return [image_url_to_base64(url) for url in image_urls]
api_key = "YOUR_API_KEY"
url = "https://api.segmind.com/v1/ssd-img2img"
# Request payload
data = {
"image": image_url_to_base64("https://segmind-sd-models.s3.amazonaws.com/outputs/ssd_img2img_input.jpeg"), # Or use image_file_to_base64("IMAGE_PATH")
"prompt": "photo of beautiful age 18 girl, pink hair, beautiful, close up, young, dslr, 8k, 4k, ultrarealistic, realistic, natural skin, textured skin",
"negative_prompt": "painting, drawing, sketch, cartoon, anime, manga, render, CG, 3d, watermark, signature, label, long neck",
"samples": 1,
"scheduler": "Euler a",
"num_inference_steps": 30,
"guidance_scale": 7.5,
"seed": 452361789,
"strength": 0.9,
"base64": False
}
headers = {'x-api-key': api_key}
response = requests.post(url, json=data, headers=headers)
print(response.content) # The response is the generated image
Attributes
Input Image
Prompt to render
Prompts to exclude, eg. 'bad anatomy, bad hands, missing fingers'
Number of samples to generate.
min : 1,
max : 4
Type of scheduler.
Allowed values:
Number of denoising steps.
min : 20,
max : 100
Scale for classifier-free guidance
min : 1,
max : 25
Seed for image generation.
min : -1,
max : 999999999999999
Scale for classifier-free guidance
min : 0,
max : 0.99
Base64 encoding of the output image.
To keep track of your credit usage, you can inspect the response headers of each API call. The x-remaining-credits property will indicate the number of remaining credits in your account. Ensure you monitor this value to avoid any disruptions in your API usage.
Segmind Stable Diffusion 1B (SSD-1B) Img2Img
Segmind Stable Diffusion 1B (SSD-1B) Img2Img is a cutting-edge AI model that is reshaping the landscape of image-to-image transformations. Leveraging advanced machine learning techniques, SSD-1B excels at converting conceptual prompts into vivid visuals, refining images, and facilitating seamless image translations guided by nuanced text inputs. It stands as a pivotal tool for creatives, marketers, and software developers who are looking to break new ground in the realm of visual content creation.
At the core of SSD-1B Img2Img is a sophisticated algorithm adept at intricate visual content manipulation. With the ability to take an existing image and, through text-driven prompts, transform it into a new piece that resonates with the creator's intent, the modle shines in performing style transfers, enhancing details, and altering subjects while preserving the essence of the original image.
Advantages
-
Text-Directed Transformation: Employs text prompts to direct the transformation process, aligning the output closely with the creator's objectives .
-
Fluid Style Adaptation:Capably adjusts the style from one image to another, ensuring transitions are coherent and purposeful.
-
Enhanced Detailing:Elevates the intricacies within images, enhancing definition and vibrancy.
-
Expansive Creative Range: Provides a broad spectrum of creative possibilities, ranging from nuanced tweaks to complete conceptual overhauls.
Use Cases
-
Creative Artwork: Enables artists to push the boundaries of their work, exploring new styles and themes effortlessly.
-
Marketing Material: Assists marketers in crafting images that are in sync with brand stories, maintaining uniformity across all visual communications.
-
Product Design: Allows designers to swiftly generate multiple product iterations, accelerating the design process.
-
Entertainment Media: Supports content creators in the entertainment sector to adjust and refine visual elements to match dynamic narratives.
-
Educational Tools: Aids educators in developing bespoke visual aids that enhance the learning experience for complex subjects.
Other Popular Models
sdxl-img2img
SDXL Img2Img is used for text-guided image-to-image translation. This model uses the weights from Stable Diffusion to generate new images from an input image using StableDiffusionImg2ImgPipeline from diffusers
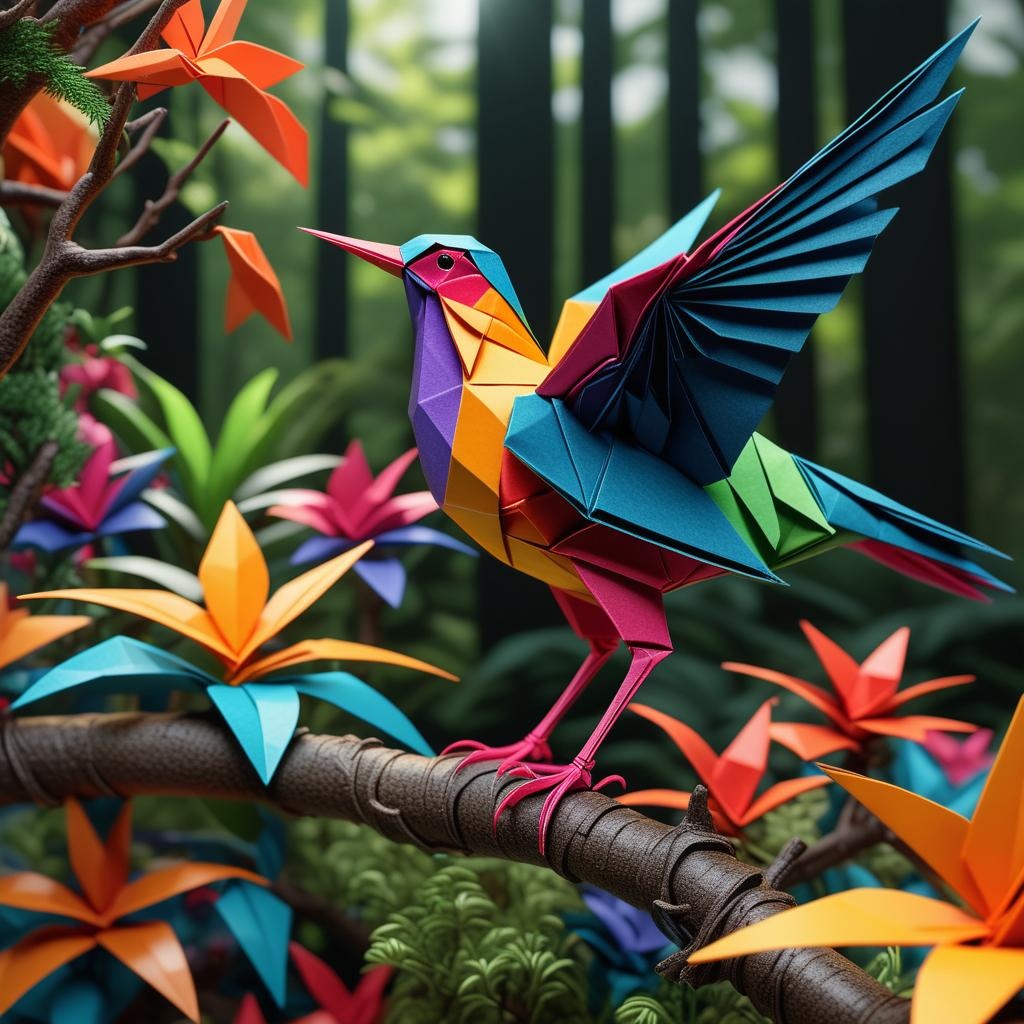
sdxl-inpaint
This model is capable of generating photo-realistic images given any text input, with the extra capability of inpainting the pictures by using a mask
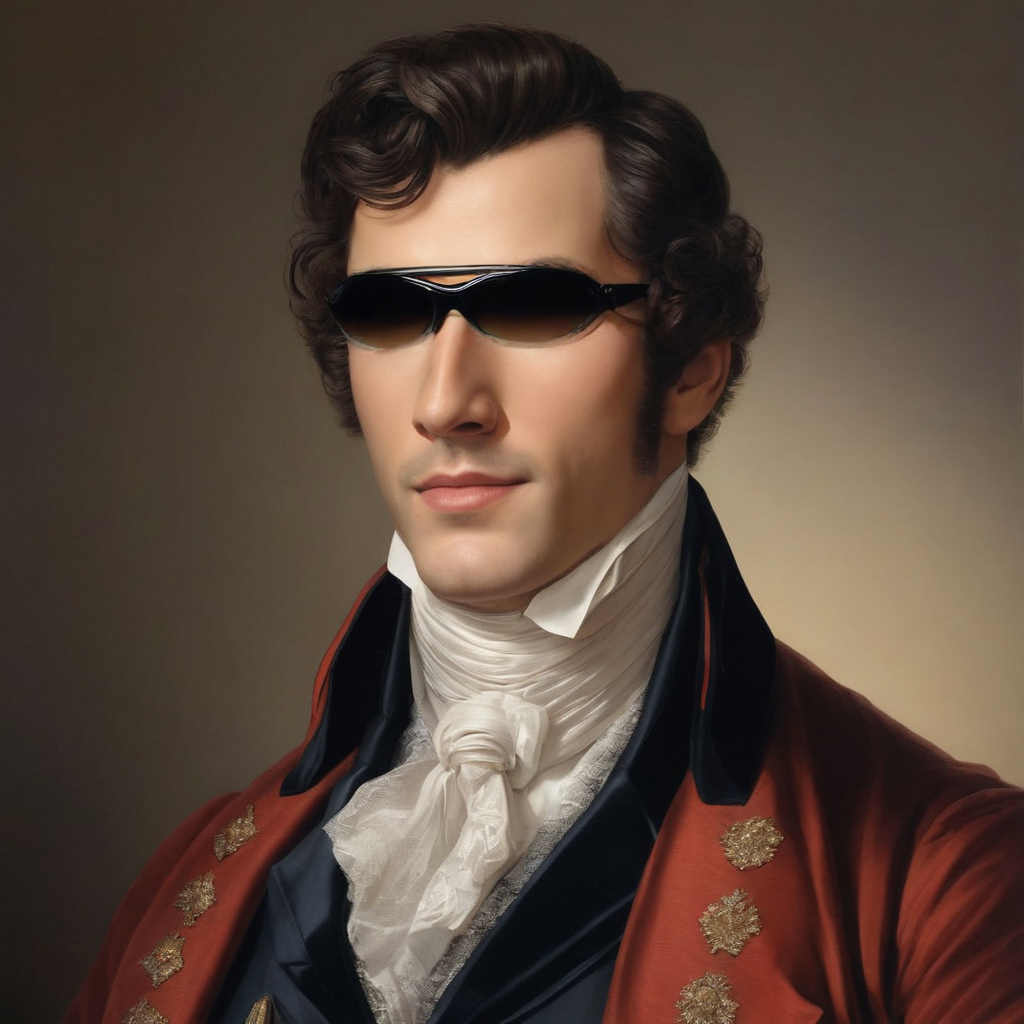
codeformer
CodeFormer is a robust face restoration algorithm for old photos or AI-generated faces.
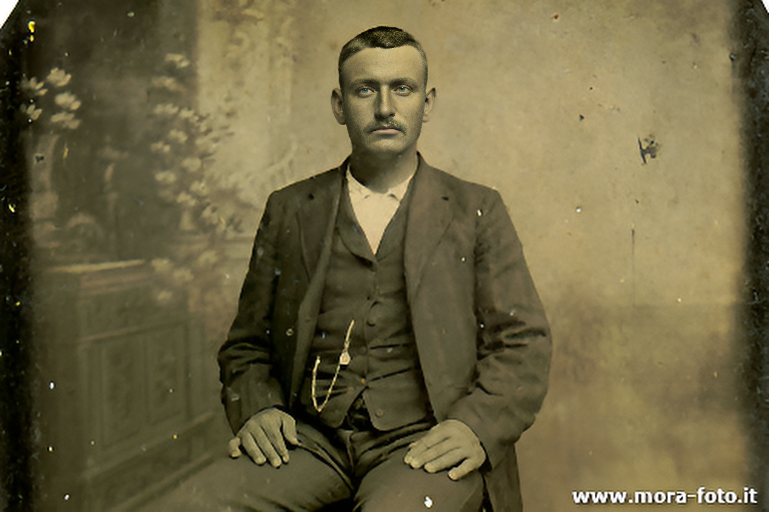
sd2.1-faceswapper
Take a picture/gif and replace the face in it with a face of your choice. You only need one image of the desired face. No dataset, no training
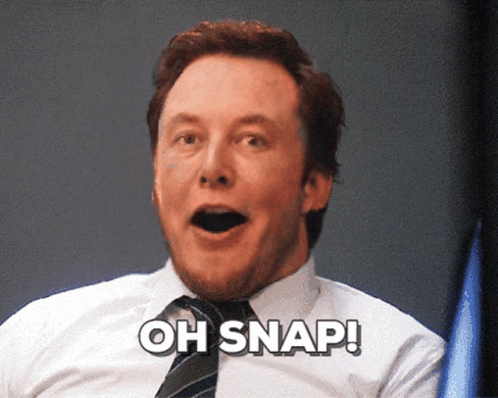