API
If you're looking for an API, you can choose from your desired programming language.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
import requests
import base64
# Use this function to convert an image file from the filesystem to base64
def image_file_to_base64(image_path):
with open(image_path, 'rb') as f:
image_data = f.read()
return base64.b64encode(image_data).decode('utf-8')
# Use this function to fetch an image from a URL and convert it to base64
def image_url_to_base64(image_url):
response = requests.get(image_url)
image_data = response.content
return base64.b64encode(image_data).decode('utf-8')
api_key = "YOUR_API_KEY"
url = "https://api.segmind.com/v1/video-loop"
# Request payload
data = {
"input_video": "https://segmind-resources.s3.amazonaws.com/input/c08771b9-b671-4c12-9ea7-af4048b9d194-894a8bdf-6064-40ea-a78d-06c1abff262b.mp4",
"loop_count": 2,
"loop_type": "normal",
"include_audio": True,
"base64": False
}
headers = {'x-api-key': api_key}
response = requests.post(url, json=data, headers=headers)
print(response.content) # The response is the generated image
Attributes
URL of the input video to be processed
Number of times to loop the video
min : 1,
max : 15
Type of loop effect to apply to the video
Allowed values:
Whether to include audio from the original video in the output
Whether to return the output video as a base64 encoded string
To keep track of your credit usage, you can inspect the response headers of each API call. The x-remaining-credits property will indicate the number of remaining credits in your account. Ensure you monitor this value to avoid any disruptions in your API usage.
Video Loop
The Video Loop effortlessly repeat video content for social media and storytelling purposes. Content creators and social media users can enhance engagement through captivating looped videos. Its key differentiator lies in the simplicity of creating both standard loops and dynamic back-and-forth motions.
Key Features
-
Effortless Video Looping - Easily repeat your video content to emphasize key moments, create seamless background visuals, or extend short clips for social media platforms.
-
Boomerang Video Effect - Generate a captivating back-and-forth motion, adding a playful and attention-grabbing element to your videos.
-
Social Media Optimization - Create engaging looped content specifically designed to capture attention and increase watch time on platforms like Instagram, TikTok, and more.
-
Enhanced Storytelling - Utilize repetition strategically to build suspense, highlight impactful scenes, or create a rhythmic flow in your narrative.
Use Cases
-
Social Media Marketing - Create repeating product demonstrations or visually appealing promotional clips that grab attention on social feeds.
-
Content Creation - Develop engaging short-form content, tutorial segments that emphasize key steps, or artistic visual loops for various online platforms.
-
Event Promotion - Showcase highlights from events in a continuous loop to generate excitement and share key moments effectively.
-
Personal Expression - Create fun and dynamic boomerang videos for social sharing, adding a unique touch to personal content.
The Video Loop offers a simple yet powerful way to transform your videos with the engaging power of repetition and the fun of boomerang effects. Enhance your social media content and storytelling effortlessly.
Other Popular Models
faceswap-v2
Take a picture/gif and replace the face in it with a face of your choice. You only need one image of the desired face. No dataset, no training
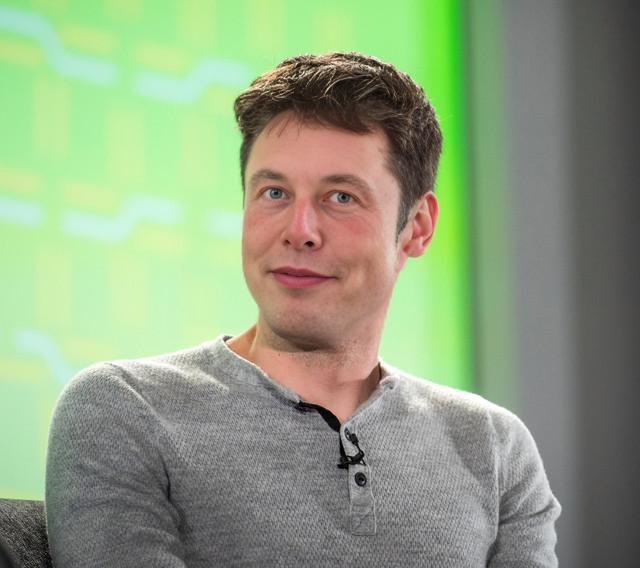
sdxl1.0-txt2img
The SDXL model is the official upgrade to the v1.5 model. The model is released as open-source software
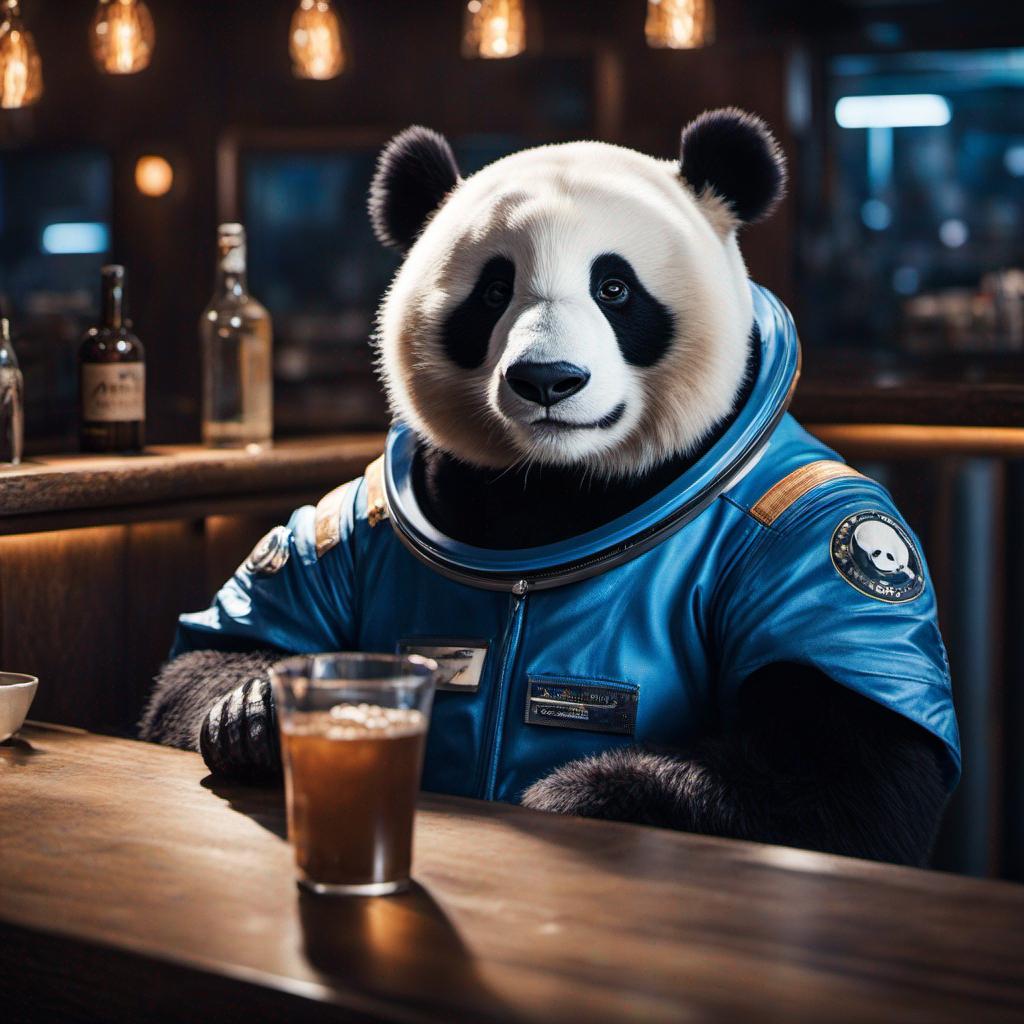
codeformer
CodeFormer is a robust face restoration algorithm for old photos or AI-generated faces.
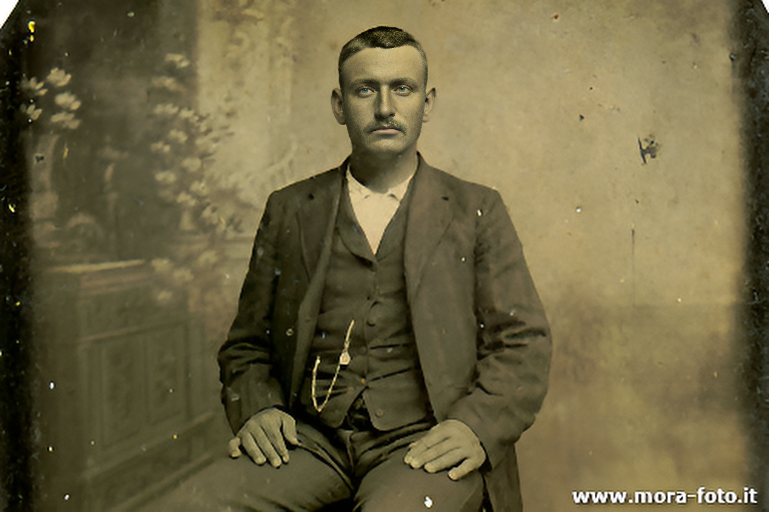
sd2.1-faceswapper
Take a picture/gif and replace the face in it with a face of your choice. You only need one image of the desired face. No dataset, no training
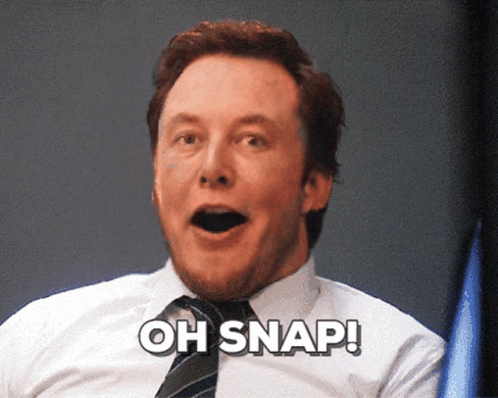