API
If you're looking for an API, you can choose from your desired programming language.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
import requests
import base64
# Use this function to convert an image file from the filesystem to base64
def image_file_to_base64(image_path):
with open(image_path, 'rb') as f:
image_data = f.read()
return base64.b64encode(image_data).decode('utf-8')
# Use this function to fetch an image from a URL and convert it to base64
def image_url_to_base64(image_url):
response = requests.get(image_url)
image_data = response.content
return base64.b64encode(image_data).decode('utf-8')
# Use this function to convert a list of image URLs to base64
def image_urls_to_base64(image_urls):
return [image_url_to_base64(url) for url in image_urls]
api_key = "YOUR_API_KEY"
url = "https://api.segmind.com/v1/esrgan-video-upscaler"
# Request payload
data = {
"crop_to_fit": False,
"input_video": "https://segmind-sd-models.s3.amazonaws.com/display_images/video-upscale-input.mp4",
"res_model": "RealESRGAN_x4plus",
"resolution": "FHD"
}
headers = {'x-api-key': api_key}
response = requests.post(url, json=data, headers=headers)
print(response.content) # The response is the generated image
Attributes
Option to crop the video to fit the specified resolution
File path or URL of the input video
Upscaling model used for resolution enhancement
Allowed values:
Output resolution for the video
Allowed values:
To keep track of your credit usage, you can inspect the response headers of each API call. The x-remaining-credits property will indicate the number of remaining credits in your account. Ensure you monitor this value to avoid any disruptions in your API usage.
ESRGAN Video Upscaler: Revolutionizing Video Quality Enhancement
The ESRGAN (Enhanced Super-Resolution Generative Adversarial Networks) video upscaler is a cutting-edge AI model designed to enhance video quality by increasing resolution and reducing artifacts. ESRGAN offers a practical solution for video restoration and upscaling, making it a valuable tool for content creators, filmmakers, and video enthusiasts.ESRGAN is based on a generative adversarial network (GAN) architecture, which consists of a generator and a discriminator. The generator enhances the video resolution, while the discriminator ensures the output is realistic and high-quality.
Key Features of ESRGAN Video Upscaler
-
High-Quality Upscaling: ESRGAN excels in upscaling videos to higher resolutions while preserving intricate details and textures. This results in sharper and more visually appealing 4k videos.
-
Artifact Reduction: The model effectively reduces common video artifacts such as noise, blurriness, and compression artifacts, ensuring a cleaner output.
-
Versatile Applications: From enhancing old home videos to improving the quality of professional footage, ESRGAN is versatile and adaptable to various use cases.
Use Cases
-
Content Creation: Enhance the quality of video content for platforms like YouTube, Vimeo, and social media.
-
Film Restoration: Restore and upscale old films and videos, preserving historical footage with improved clarity.
-
Gaming: Upscale in-game footage for a more immersive and visually stunning experience.
Other Popular Models
sdxl-controlnet
SDXL ControlNet gives unprecedented control over text-to-image generation. SDXL ControlNet models Introduces the concept of conditioning inputs, which provide additional information to guide the image generation process
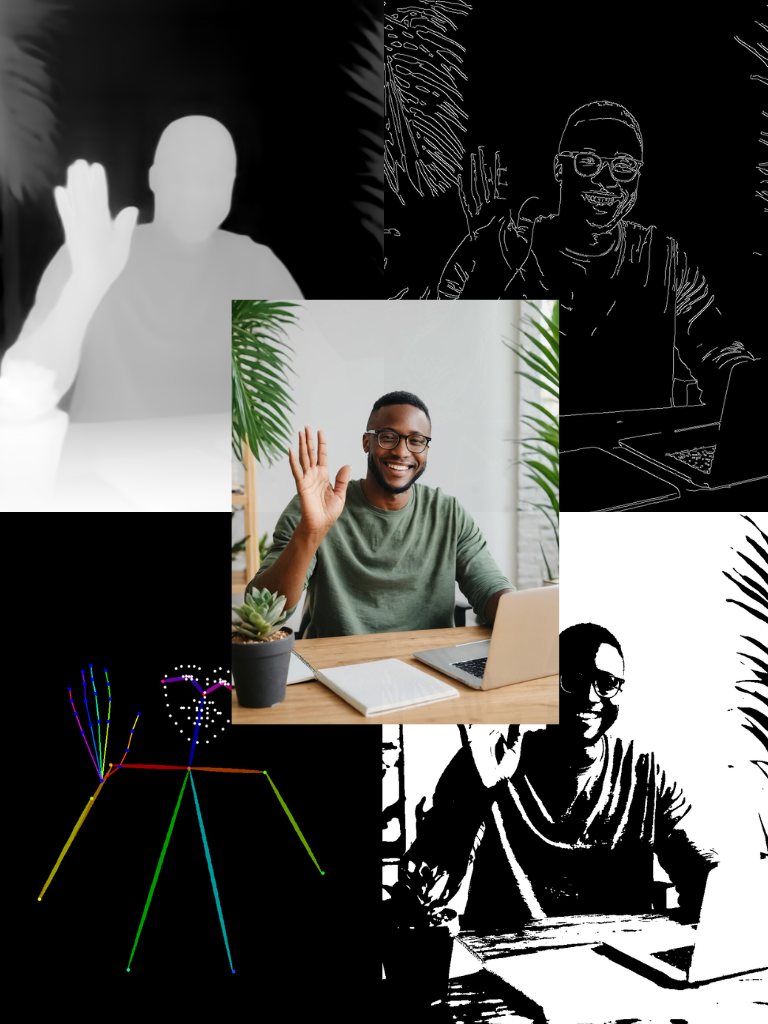
storydiffusion
Story Diffusion turns your written narratives into stunning image sequences.
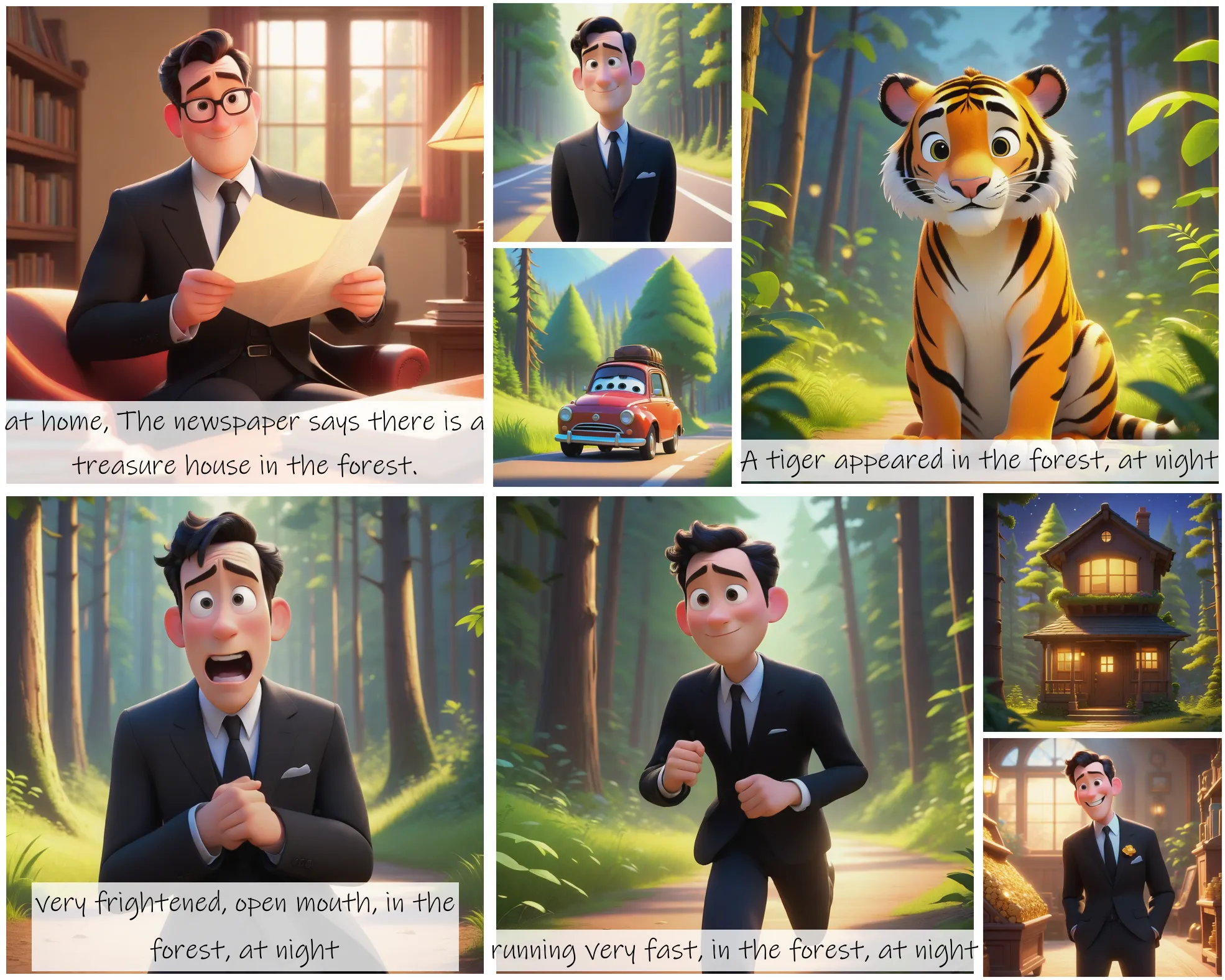
idm-vton
Best-in-class clothing virtual try on in the wild

face-to-many
Turn a face into 3D, emoji, pixel art, video game, claymation or toy
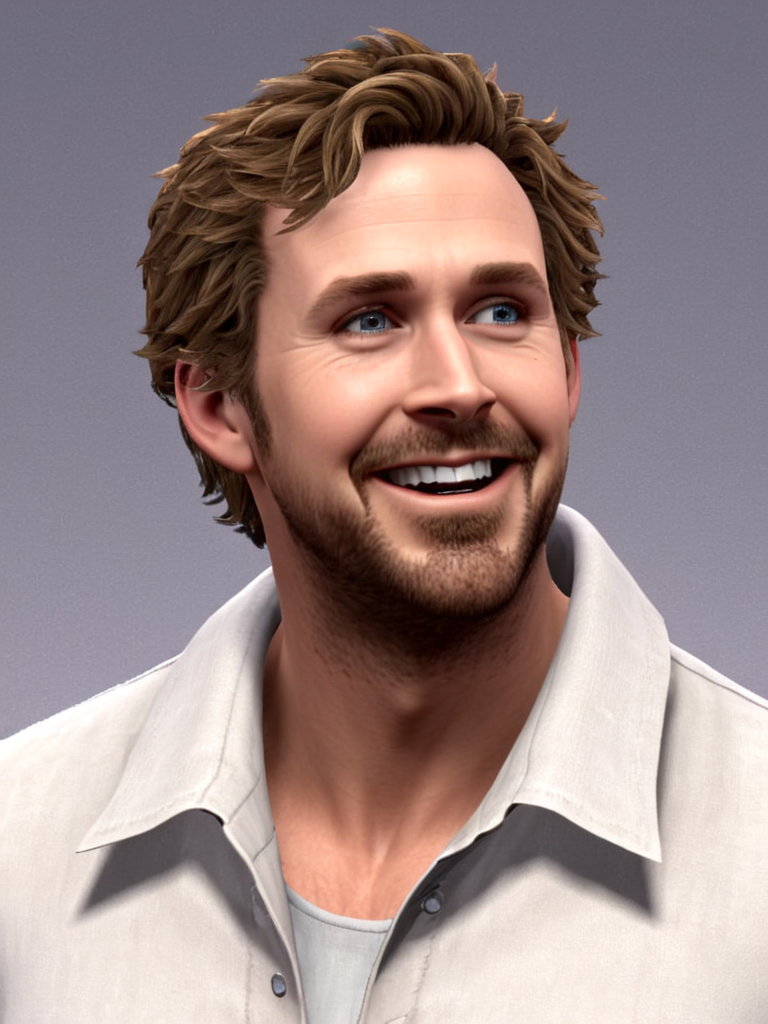