API
If you're looking for an API, you can choose from your desired programming language.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
import requests
import base64
# Use this function to convert an image file from the filesystem to base64
def image_file_to_base64(image_path):
with open(image_path, 'rb') as f:
image_data = f.read()
return base64.b64encode(image_data).decode('utf-8')
# Use this function to fetch an image from a URL and convert it to base64
def image_url_to_base64(image_url):
response = requests.get(image_url)
image_data = response.content
return base64.b64encode(image_data).decode('utf-8')
api_key = "YOUR_API_KEY"
url = "https://api.segmind.com/v1/ideogram-turbo-txt-2-img"
# Request payload
data = {
"magic_prompt_option": "AUTO",
"color_palette": {
# Either provide a named palette
"name": "MELON", # required, mutually exclusive with "members"
# Or provide custom colors
"members": [ # required, mutually exclusive with "name"
{
"color_hex": "", # required if using "members"
"color_weight": 0 # optional
}
]
},
"negative_prompt": "low quality,blurry",
"prompt": "portrait editorial, Img_4099.HEIC: cool anthropomorphic masculine tiger posing against neutral gray background, wearing alternative cyberpunk attire by Demna Gvasalia, rendered with photorealistic style, high definition, dof, create a fashion atmosphere, award winning photography, Hasselblad H6D-100c, XCD 85mm f/1.8",
"resolution": "RESOLUTION_1024_1024",
"seed": 56698,
"style_type": "GENERAL"
}
headers = {'x-api-key': api_key}
response = requests.post(url, json=data, headers=headers)
print(response.content) # The response is the generated image
Attributes
Option for magic prompt
Allowed values:
Negative prompt to avoid specific elements
Prompt to render
Resolution of the output image
Allowed values:
Seed for random generation
min : 1,
max : 99999
Style type for the output
Allowed values:
To keep track of your credit usage, you can inspect the response headers of each API call. The x-remaining-credits property will indicate the number of remaining credits in your account. Ensure you monitor this value to avoid any disruptions in your API usage.
Ideogram Turbo Text-to-Image
Ideogram Turbo text to image model designed for rapid image creation, particularly useful for quick ideation and generating images with text. Ideal for designers, marketers, and anyone needing fast visuals, it balances speed and quality, producing images in seconds. This model is especially suited for those seeking a "sketchy" or quickly rendered aestheti
Key Features
-
Turbo Rendering: Generates images in approximately 7 to 12 seconds, allowing for quick visual exploration and fast iterations.
-
Text Rendering: Excels at incorporating text seamlessly and creatively within generated images.
-
Ideation and Composition: Well-suited for quickly visualizing concepts and exploring different compositional elements.
-
"Sketchy" Aesthetic: Capable of producing images with a unique, hand-drawn or draft-like appearance
Use Cases
-
Rapid Prototyping for Design: Quickly visualize design concepts and iterate on ideas for logos, posters, and marketing materials.
-
Social Media Content Creation: Generate eye-catching visuals with integrated text for social media campaigns and posts in a matter of seconds.
-
Storyboarding and Concept Art: Create quick sketches and scene mockups for film, animation, and game development.
-
Presentations and Visual Aids: Produce illustrative images and diagrams with clear text labels for presentations and educational materials.
Other Popular Models
face-to-many
Turn a face into 3D, emoji, pixel art, video game, claymation or toy
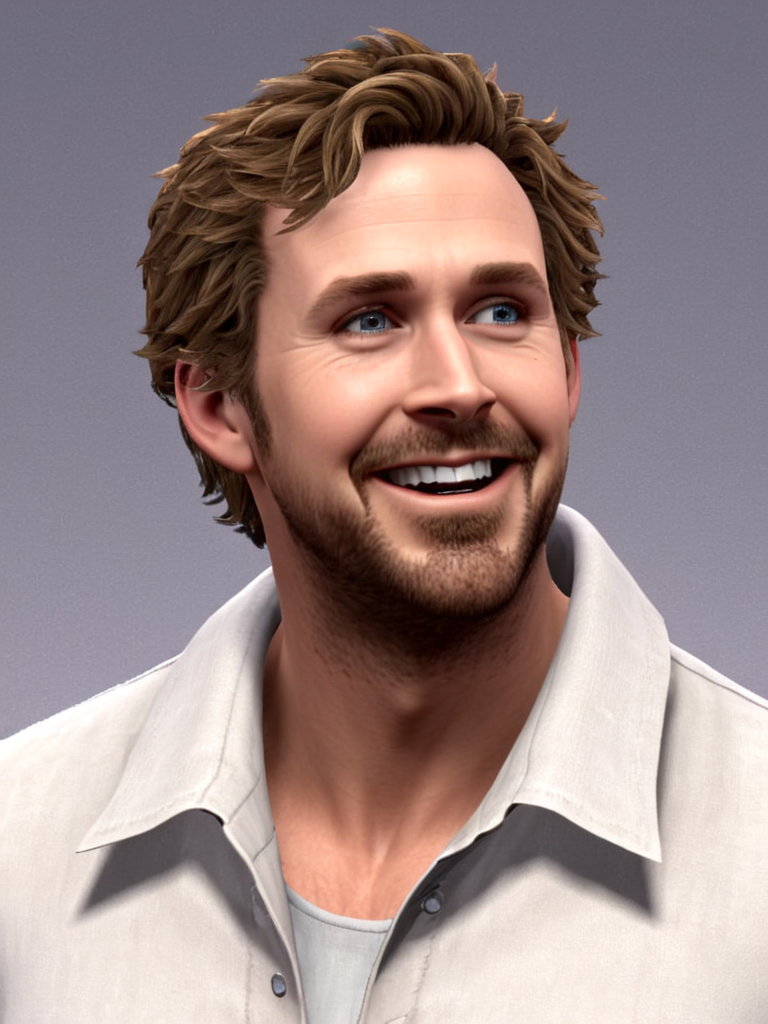
insta-depth
InstantID aims to generate customized images with various poses or styles from only a single reference ID image while ensuring high fidelity
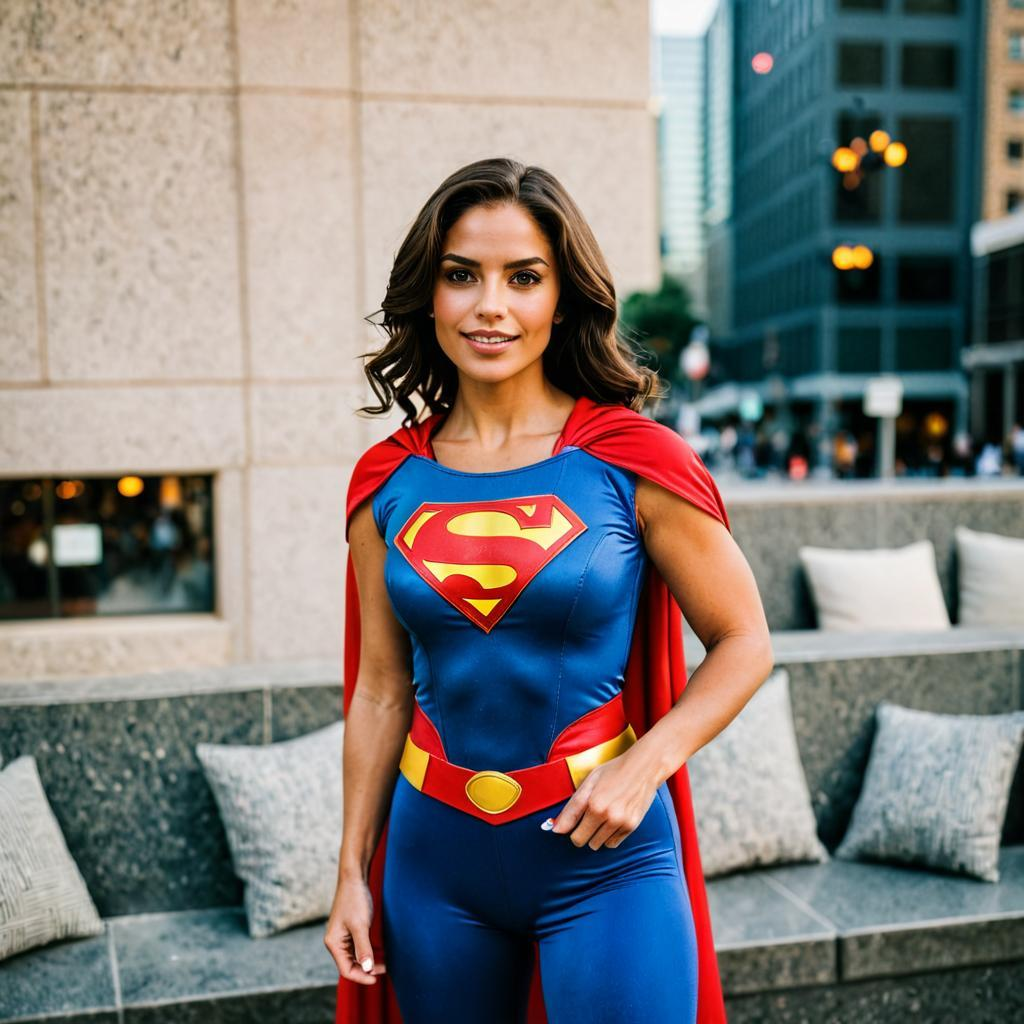
sdxl1.0-txt2img
The SDXL model is the official upgrade to the v1.5 model. The model is released as open-source software
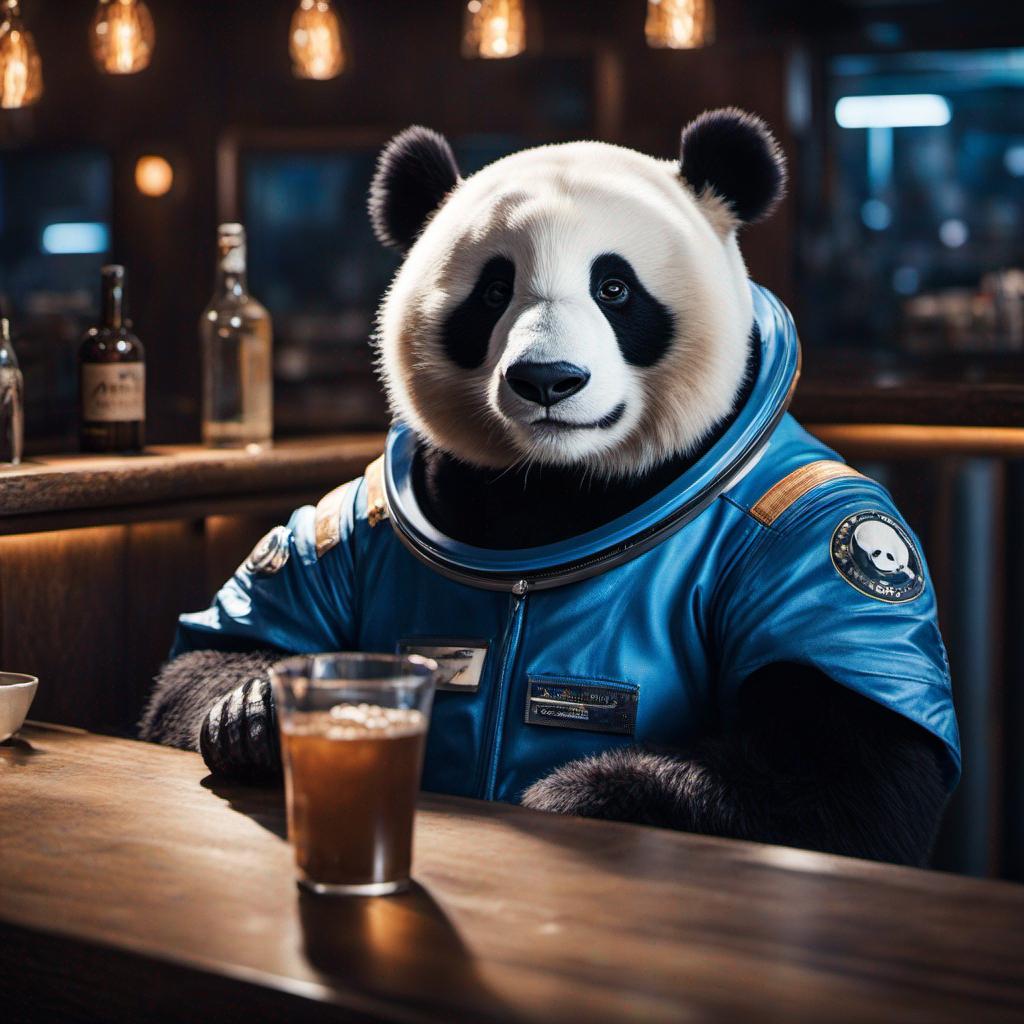
codeformer
CodeFormer is a robust face restoration algorithm for old photos or AI-generated faces.
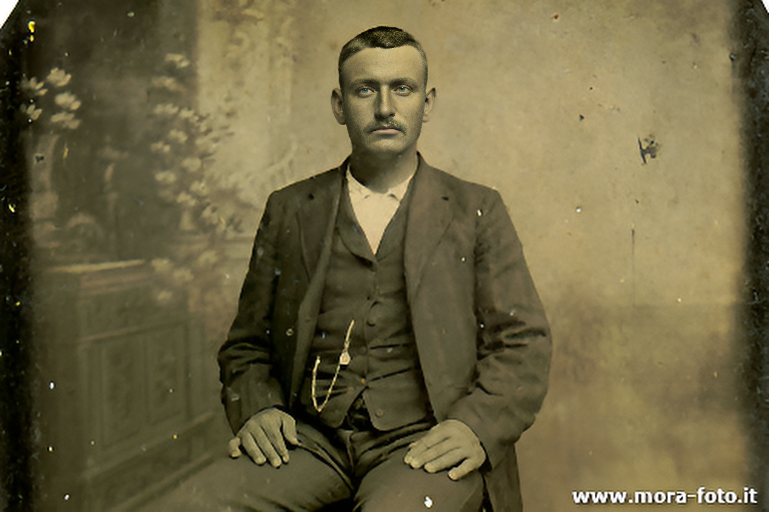