API
If you're looking for an API, you can choose from your desired programming language.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
import requests
import base64
# Use this function to convert an image file from the filesystem to base64
def image_file_to_base64(image_path):
with open(image_path, 'rb') as f:
image_data = f.read()
return base64.b64encode(image_data).decode('utf-8')
# Use this function to fetch an image from a URL and convert it to base64
def image_url_to_base64(image_url):
response = requests.get(image_url)
image_data = response.content
return base64.b64encode(image_data).decode('utf-8')
api_key = "YOUR_API_KEY"
url = "https://api.segmind.com/v1/minimaxir-sdxl-ugly-sonic-lora"
# Request payload
data = {
"prompt": "sonic the hedgehog",
"negative_prompt": "boring, poorly drawn, bad artist, (worst quality:1.4), simple background, uninspired, (bad quality:1.4), monochrome, low background contrast, background noise, duplicate, crowded, (nipples:1.2), big breasts",
"scheduler": "UniPC",
"num_inference_steps": 25,
"guidance_scale": 8,
"samples": 1,
"seed": 3426017487,
"img_width": 1024,
"img_height": 1024,
"base64": False,
"lora_scale": 1
}
headers = {'x-api-key': api_key}
response = requests.post(url, json=data, headers=headers)
print(response.content) # The response is the generated image
Attributes
Prompt to render
Prompts to exclude, eg. 'bad anatomy, bad hands, missing fingers'
Type of scheduler.
Allowed values:
Number of denoising steps.
min : 20,
max : 100
Scale for classifier-free guidance
min : 0.1,
max : 25
Number of samples to generate.
min : 1,
max : 4
Seed for image generation.
Width of the image.
Allowed values:
Height of the Image
Allowed values:
Base64 encoding of the output image.
Scale of the lora
To keep track of your credit usage, you can inspect the response headers of each API call. The x-remaining-credits property will indicate the number of remaining credits in your account. Ensure you monitor this value to avoid any disruptions in your API usage.
SDXL Ugly Sonic LoRA
Introducing the SDXL Ugly Sonic LoRA, fine tuned on images from the movie "Ugly Sonic" from the original 1080p trailer to life in stunning detail. This model is a must-have for fans, artists, and creators looking to explore the quirky and iconic version of one of the most beloved movie characters.
Advantages
-
Optimized for Creativity: Ideal for generating high-quality images of "Ugly Sonic" in various scenarios and styles.
-
Easy Trigger Keywords:imply use "sonic the hedgehog" to activate the model and start creating.
-
Optimized for Creativity:: Ideal for generating high-quality images of "Ugly Sonic" in various scenarios and styles.
Use Cases
-
Fan Art Creation:Generate unique and high-quality images of "Ugly Sonic" for fan art projects.
-
Animation and Gaming: Explore alternative character designs for animations or indie game projects.
-
Marketing and Promotions: Create eye-catching visuals for marketing campaigns, especially for retro gaming and nostalgia-themed events.
-
Personal Projects: Ideal for personal projects or social media content, especially for fans of the Sonic franchise.
Other Popular Models
sdxl-controlnet
SDXL ControlNet gives unprecedented control over text-to-image generation. SDXL ControlNet models Introduces the concept of conditioning inputs, which provide additional information to guide the image generation process
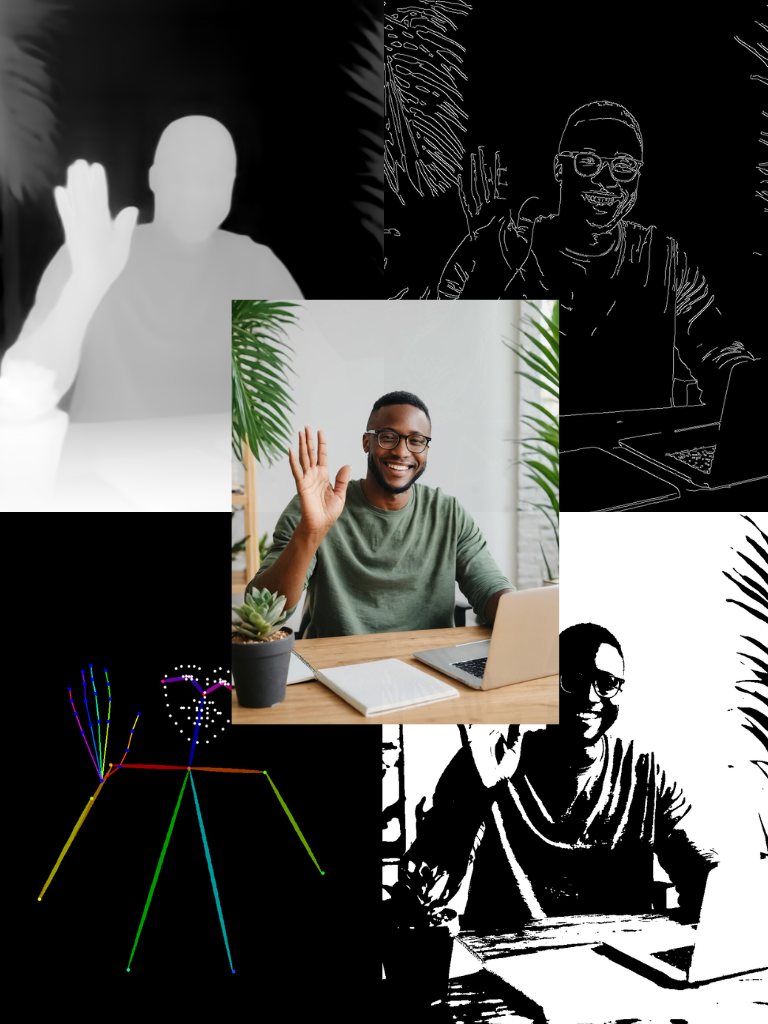
face-to-many
Turn a face into 3D, emoji, pixel art, video game, claymation or toy
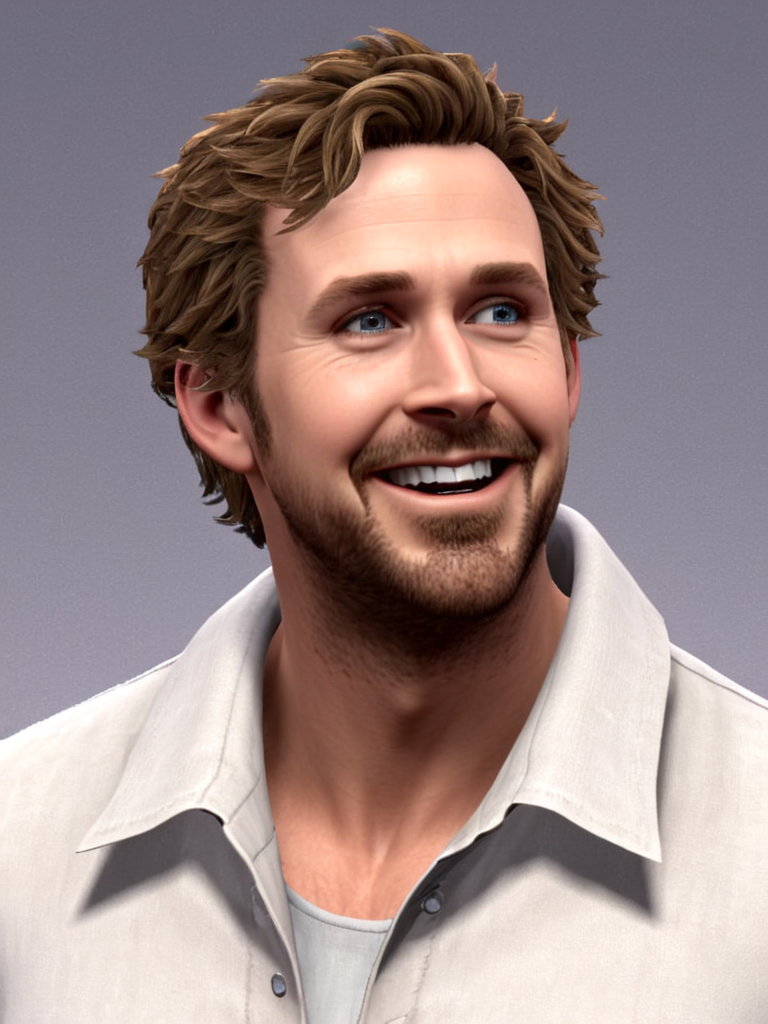
faceswap-v2
Take a picture/gif and replace the face in it with a face of your choice. You only need one image of the desired face. No dataset, no training
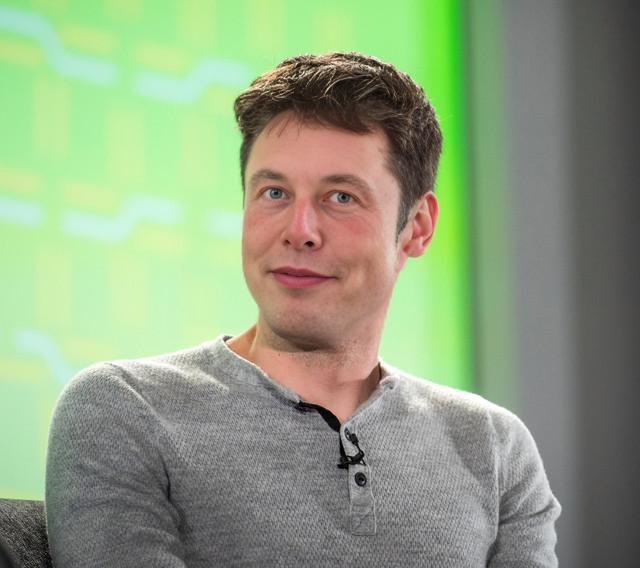
sdxl1.0-txt2img
The SDXL model is the official upgrade to the v1.5 model. The model is released as open-source software
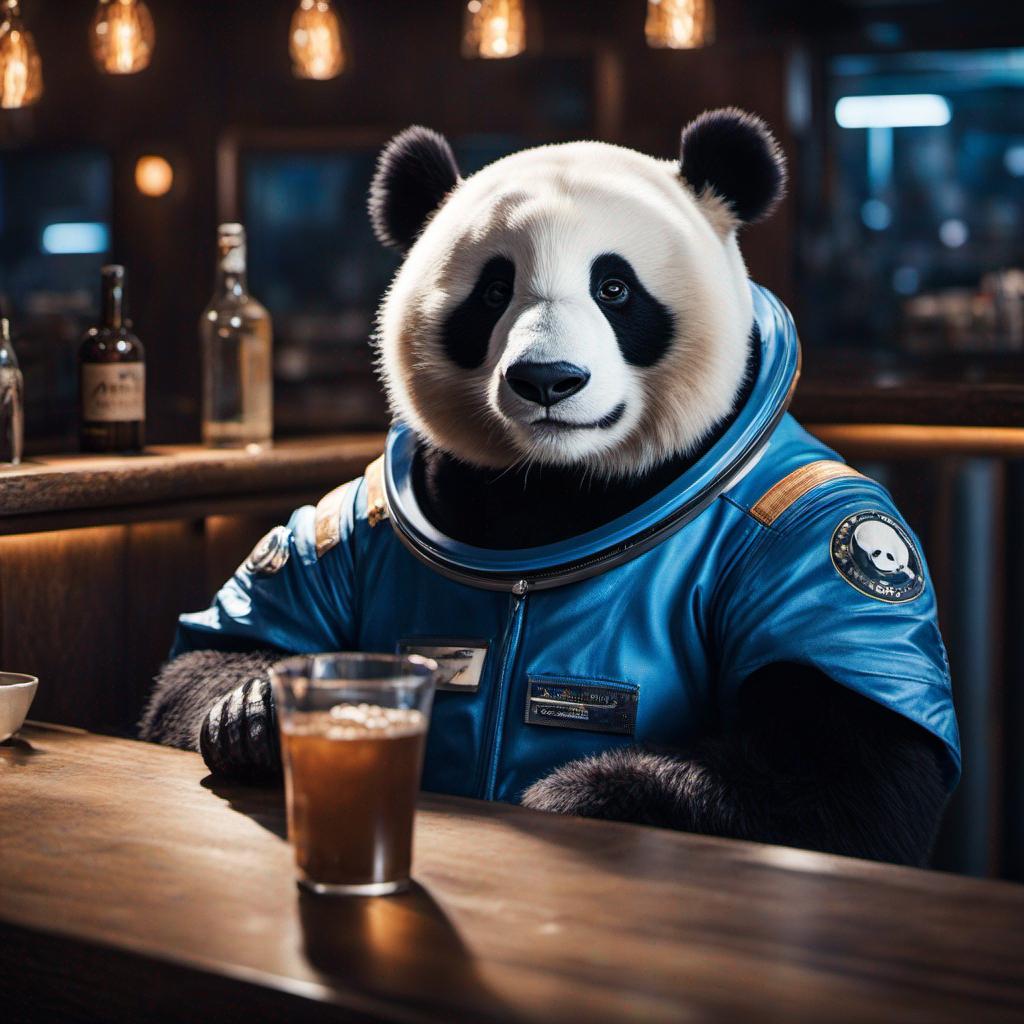