API
If you're looking for an API, you can choose from your desired programming language.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
import requests
import base64
# Use this function to convert an image file from the filesystem to base64
def image_file_to_base64(image_path):
with open(image_path, 'rb') as f:
image_data = f.read()
return base64.b64encode(image_data).decode('utf-8')
# Use this function to fetch an image from a URL and convert it to base64
def image_url_to_base64(image_url):
response = requests.get(image_url)
image_data = response.content
return base64.b64encode(image_data).decode('utf-8')
# Use this function to convert a list of image URLs to base64
def image_urls_to_base64(image_urls):
return [image_url_to_base64(url) for url in image_urls]
api_key = "YOUR_API_KEY"
url = "https://api.segmind.com/v1/superimpose-v2"
# Request payload
data = {
"base_image": image_url_to_base64("https://segmind-sd-models.s3.amazonaws.com/display_images/superimpose-v2-model.jpeg"), # Or use image_file_to_base64("IMAGE_PATH")
"overlay_image": image_url_to_base64("https://segmind-sd-models.s3.amazonaws.com/display_images/tshirt-logo-2.png"), # Or use image_file_to_base64("IMAGE_PATH")
"rescale_factor": 0.4,
"resize_method": "nearest",
"bg_remove_model": "Bria",
"mirror": "None",
"blend_mode": "screen",
"opacity": 90,
"x_percent": 50,
"y_percent": 65,
"rotation": 0,
"base64": False
}
headers = {'x-api-key': api_key}
response = requests.post(url, json=data, headers=headers)
print(response.content) # The response is the generated image
Attributes
Base image for the model
Overlay image for the model
Mask for the overlay image
Rescale factor for the overlay image
min : 0,
max : 100
Resize Method for Overlay Image.
Allowed values:
BG Removal Model
Allowed values:
Mirror
Allowed values:
Blend Mode
Allowed values:
Opacity of the Overlay Image
min : 0,
max : 100
X Percent
min : 0,
max : 100
Y Percent
min : 0,
max : 100
Rotation
Allowed values:
min : 0,
max : 360
Base64 encoding of the output image.
To keep track of your credit usage, you can inspect the response headers of each API call. The x-remaining-credits property will indicate the number of remaining credits in your account. Ensure you monitor this value to avoid any disruptions in your API usage.
Superimpose V2
Superimpose V2 allows you to easily create captivating visuals by seamlessly overlaying one image on top of another. You have various ways to resize the overlay image, including maintaining its proportions, setting specific dimensions, or automatically fitting it to the base image. Additionally, you can choose from different resizing methods to achieve smooth transitions, exceptional quality, or faster processing depending on your needs. Finally, precise positioning tools let you perfectly align the overlay image on the base image. This is an improvement over Superimpose v1 to make image editing more seamless than before.
What’s new in Superimpose V2
-
Background removal is baked into the model, to create transparent background images. No need to upload pre-existing transparent images like Superimpose v1.
-
The blending mode specified in the options bar controls how pixels in the image are affected by superimposition. There are 14 blending modes present. Think in terms of the following colors when visualizing a blending mode’s effect:
-
The base color is the original color in the image.
-
The blend color is the color being applied with the superimposition.
-
The result color is the color resulting from the blend.
Other Popular Models
face-to-many
Turn a face into 3D, emoji, pixel art, video game, claymation or toy
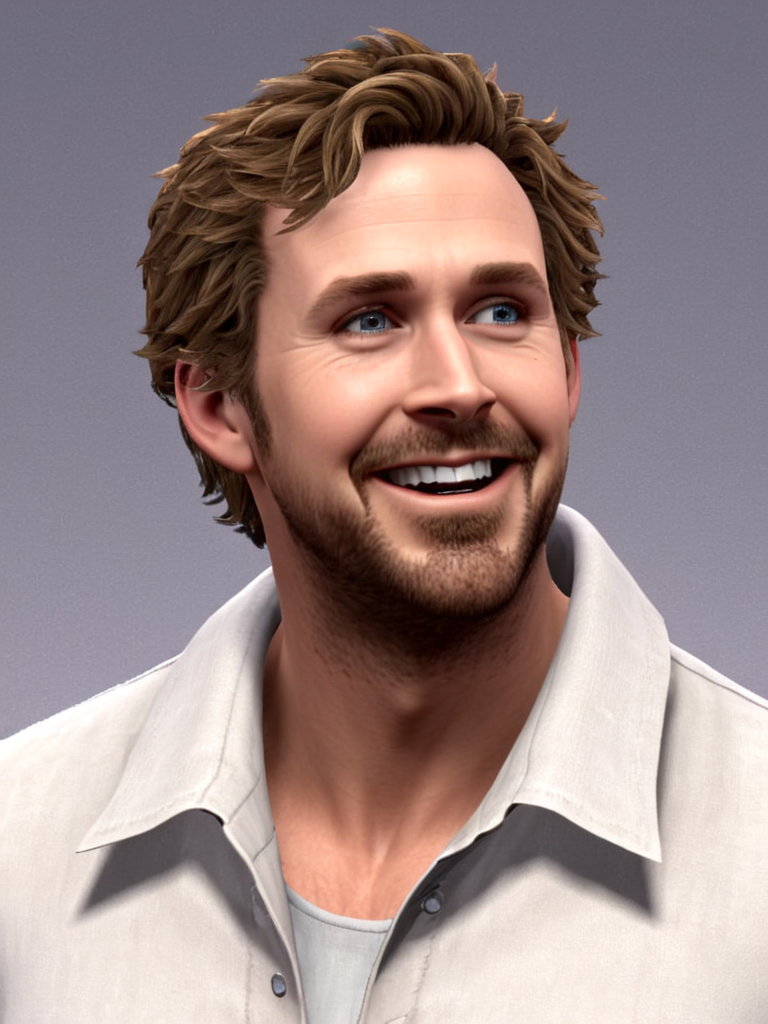
faceswap-v2
Take a picture/gif and replace the face in it with a face of your choice. You only need one image of the desired face. No dataset, no training
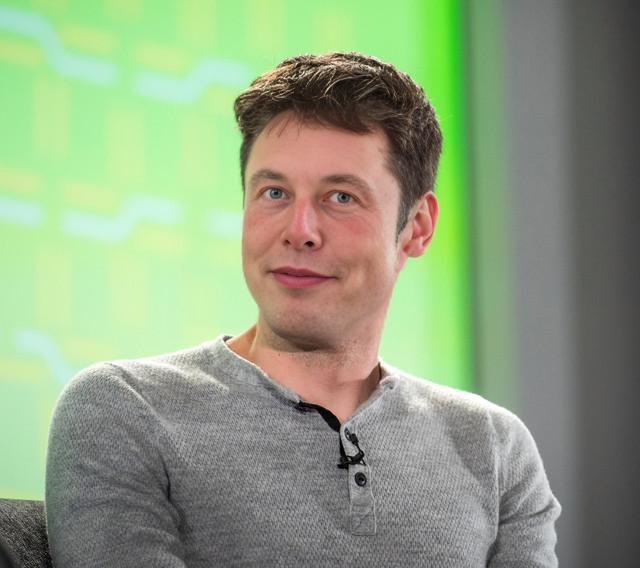
sdxl1.0-txt2img
The SDXL model is the official upgrade to the v1.5 model. The model is released as open-source software
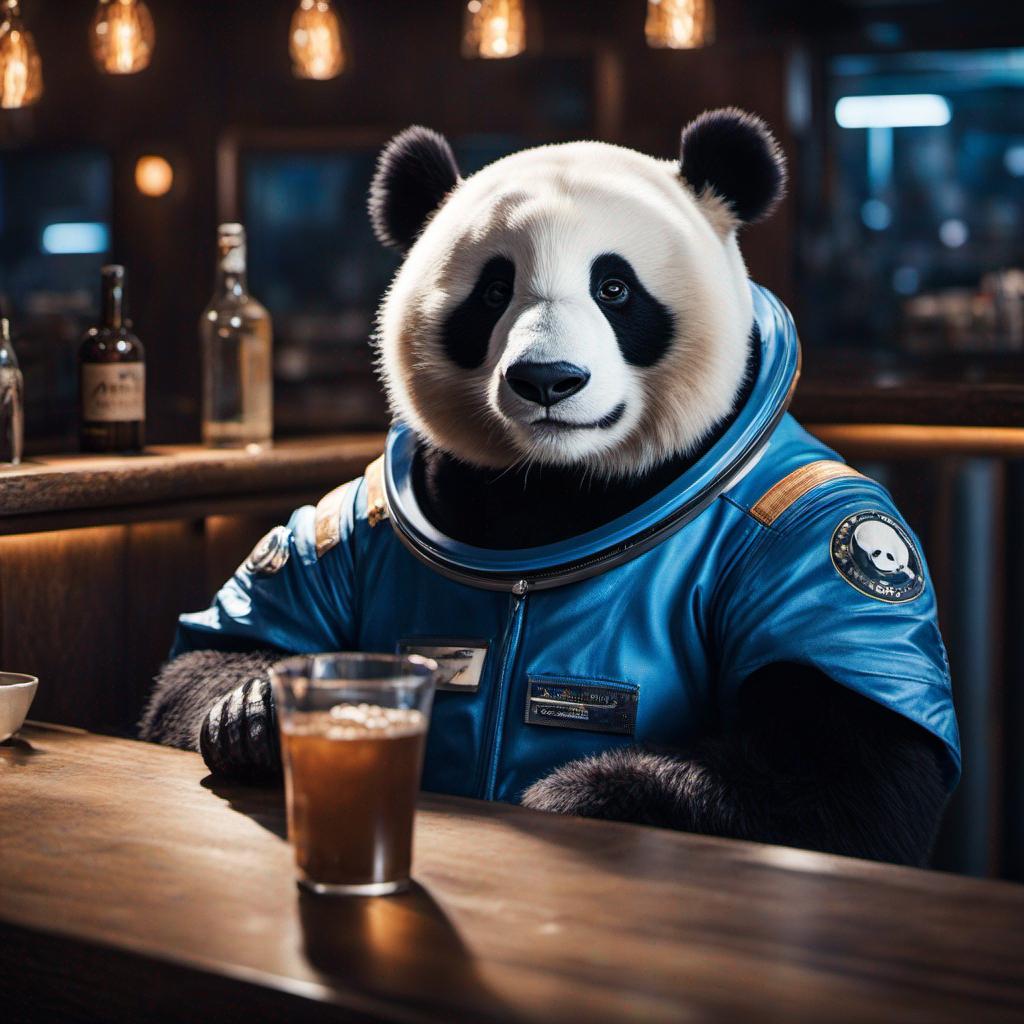
sd2.1-faceswapper
Take a picture/gif and replace the face in it with a face of your choice. You only need one image of the desired face. No dataset, no training
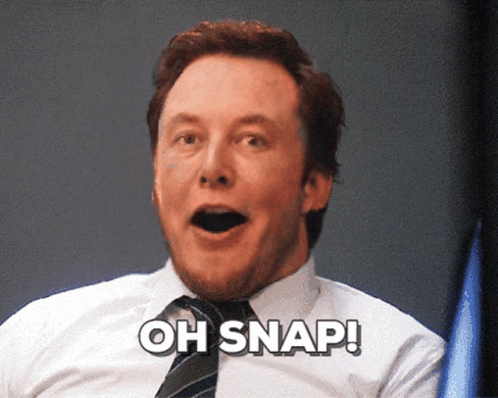